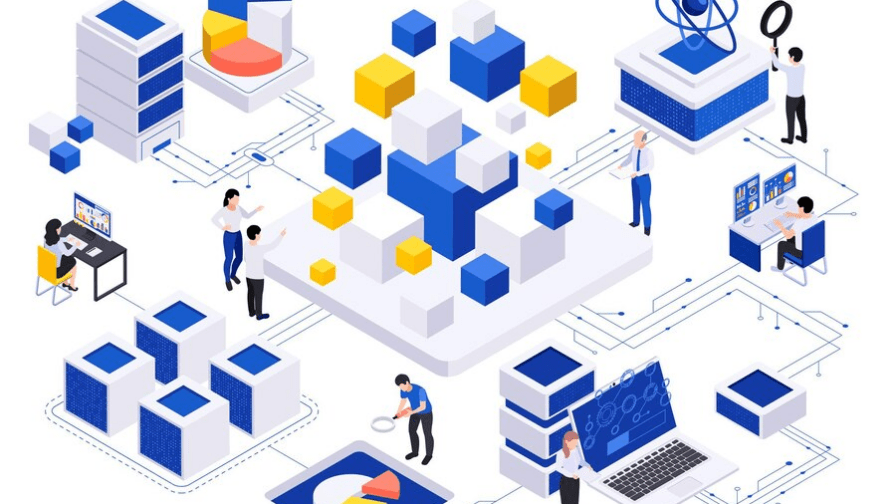
Microservices have taken the software industry by storm, offering a new architectural style that breaks applications into smaller, more manageable pieces. This provides improved flexibility, scalability, and maintainability for software applications. As a versatile and widely adopted programming language, Python has naturally found its place in the microservices world. With its rich ecosystem, clean syntax, and robust frameworks, Python emerges as a leading choice for building efficient microservices.
In this article, we cover the benefits of using Python for microservices and provide the general steps you’ll need to go through to build, deploy, and test microservices using Python.
Recommended article: Automate everything with Python
Advantages of Python for Microservices Development
Ease of Use and Readability
Python’s syntax is designed to be easy to understand and write, which makes it an excellent choice for developers of all skill levels. This simplicity doesn’t come at the expense of functionality; Python is a powerful language that can handle complex tasks with ease.
Python’s readability also makes it ideal for microservices development. In a microservices architecture, you’re likely dealing with a large number of services developed by multiple teams. If the codebase is difficult to read and understand, maintaining your system can become a nightmare.
Extensive Libraries and Integrations
Another advantage of Python for microservices development is the extensive range of libraries and integrations it offers. Python’s standard library is well-stocked with modules that can help you perform a variety of tasks, from data serialization to network communication. Plus, the Python community has created a vast array of third-party libraries that you can use to extend Python’s capabilities even further.
Python’s integrations with other technologies are also a big plus. Whether you need to interact with a database, a message queue, or an external API, Python likely has a library or tool that can help. This interoperability can be a significant advantage when building microservices, as you may need to integrate your services with a wide variety of other systems.
Mature RESTful API Development Tools
When it comes to building microservices, you’ll often need to provide a RESTful API for your services to communicate with each other. Python shines in this area thanks to its mature tools for RESTful API development. Frameworks like Flask and Django provide robust support for building APIs, including features like request routing, data validation, and error handling.
These tools also make it easier to follow best practices for API development. For example, they often encourage the use of HTTP status codes to indicate the outcome of a request, which can make your API easier to use and understand. Plus, many Python frameworks support the development of self-documenting APIs, which can be a huge help when you’re working with a large number of services.
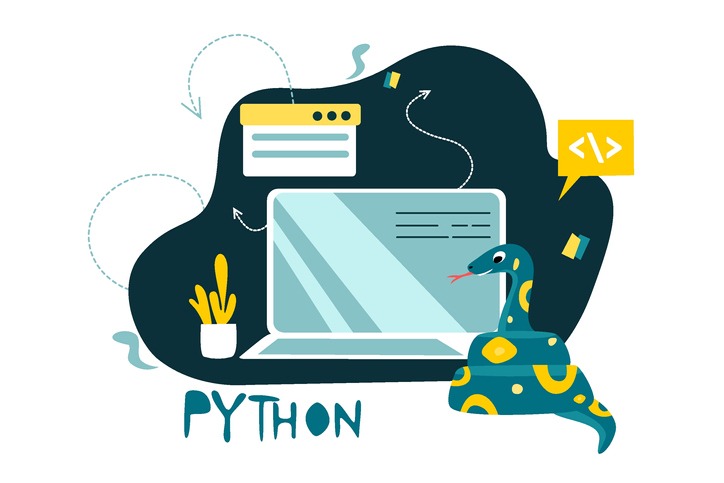
Steps for Building Microservices in Python
Setting Up the Python Environment
Before we can start building our microservices, we’ll need to set up our Python environment. Start by installing Python on your machine if you haven’t already. Then, create a virtual environment for your project. A virtual environment is a self-contained Python environment that you can use to keep your project’s dependencies separate from your system’s Python installation.
Next, install the libraries and tools you’ll need for your project. This might include a web framework like Flask or Django, a library for making HTTP requests, a database driver, and so on. You can install these libraries using pip, Python’s package manager.
Selecting a Microservice Framework
The next step is to choose a framework for your microservices. There are several good options to choose from, each with its own strengths and weaknesses. Flask is a lightweight framework that gives you a lot of flexibility but may require you to do more work up front. Django is a more full-featured framework that can do a lot of the heavy lifting for you, but it might be overkill for a simple microservice.
Another option is to use a framework specifically designed for microservices, like Nameko or PyMS. These frameworks provide features like service discovery, load balancing, and fault tolerance out of the box, which can make your life a lot easier if you’re building a complex system.
Recommended video: Writing Async Microservices in Python
Designing Your Microservices
Once you’ve chosen a framework, it’s time to start designing your microservices. This is where you’ll decide what each service will do, how they’ll communicate with each other, and how they’ll handle errors.
When designing your services, try to follow the principle of single responsibility. Each service should have one job and do it well. This will make your services easier to understand, test, and maintain.
Building a Simple Microservice
Now we’re ready to start coding. Let’s begin by building a simple microservice. Let’s say we’re building a system for a library, and our first service is responsible for managing books. We might start by defining routes for creating, retrieving, updating, and deleting books.
Once we’ve got our routes defined, we can start implementing the logic for each route. This might involve interacting with a database, validating input data, or calling other services.
Running Microservices App in Kubernetes
After we’ve built our microservice, we need to run it. One popular way to run microservices is deploying them with Kubernetes, an open-source platform for managing containerized applications.
To run our microservice in Kubernetes, we’ll first need to containerize it. We can do this using a tool like Docker. Once our service is containerized, we can deploy it to a Kubernetes cluster. You can run Kubernetes clusters on-premises or in a cloud provider like AWS.
Kubernetes provides a lot of features that can help us manage our microservices, like service discovery, load balancing, and automatic scaling. It can also help us ensure that our services are always up and running, even if some of our servers go down.
Testing Microservices in Python
Finally, we need to test our microservices. Testing is crucial in a microservices architecture, as it can help us catch bugs before they cause problems in production.
Python provides several tools for testing, like the unittest module in the standard library and third-party libraries like pytest. These tools can help us write tests for our services and run them automatically.
When testing microservices, it’s important to test not only the individual services but also their interactions. This can be challenging, as it requires us to set up a realistic testing environment and simulate the behavior of other services. But it’s crucial for ensuring that our system works correctly as a whole.
In conclusion, Python offers several key advantages for microservices development, including ease of use, extensive libraries and integrations, and mature RESTful API development tools. By following the steps outlined in this article, you can leverage these advantages to build robust, scalable microservices in Python.