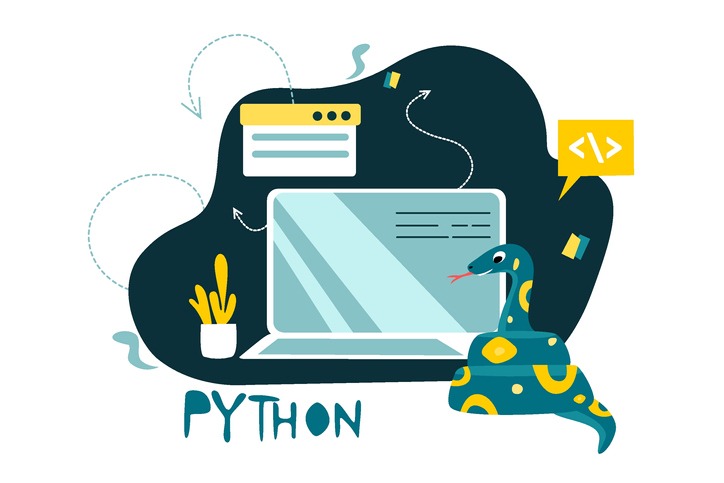
Did you know that there are currently around 4,294,967,296 IPv4 addresses in use?
That’s a big number.
So big, in fact, that a new standard is being introduced to cope with demand: IPv6.
In this article, we’re going to explore how to validate an IP address using Python. This can be useful for all sorts of scenarios, whether you’re working on fraud prevention or on more general projects like developing an iPaaS platform (you can find an iPaaS definition here).
But first, let’s look in more detail at the differences between the IPv4 and IPv6 IP address formats. When it comes to IP address validation, you may need to tweak your code to take account of these variations.
What’s the difference between IPv4 and IPv6?
Imagine, if you will, the very early days of the internet, before things like cloud phone systems were even a twinkle in a telecoms executive’s eye. The very first engineers working on IP (internet Protocol) invented IPv4, which – despite the “version 4” bit – was actually the first internet protocol introduced and is still in use today.
You will of course be very familiar with the format, which is four numbers between 0 and 255 separated by periods, like this: 38.251.131.135
Each number is an 8-bit field and represents a single byte of the IP address. That means that the complete IP is a 32-bit (4 x 8 bits) address space. Altogether, this allows for enough combinations to generate 4.3 billion unique addresses.
Now, back when those early engineers were coming up with this concept, they couldn’t have foreseen the scale of the modern internet or imagined that tens of millions of devices might want to access a single co.nz domain at once. Quite understandably, they assumed that 4.3 billion addresses would be more than enough.
Well, as we saw at the beginning of this article, we’ve already nearly reached that.
Fortunately, some smart people noticed this issue coming down the tracks years ago and set up the Internet Engineering Task Force (IETF). Back in 1998, this august body introduced IPv6, which instead uses 128-bit addressing. This means it can be used to support around 340 trillion trillion addresses. So hopefully, it will be a long time before we run out of IPv6 capacity. Tasks like VoIP migration should be easily achievable for many years to come.
IPv6 format is eight hexadecimal numbers separated by colons and looks like this:
2510:cc:8000:1c82:543c:cd2e:f2fa:5a8b
In this article we’ll be focusing on validating IPv4 addresses, but the same principles we cover here are applicable to IPv6 addresses too.
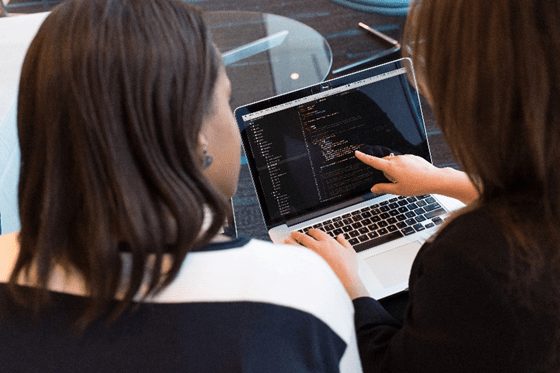
Free to use image from Unsplash
Validating an IP address with Python
Python is an object-oriented and dynamically typed coding language well loved by amateur coders and senior developers alike. Because it’s so flexible and scalable, it can be used for all sorts of applications. From using Python for automation to developing websites and data visualisation tools, it’s a terrific option for accomplishing varied technical tasks.
There are a number of ways of validating IP addresses in Python. Here we present two of the most straightforward methods: using the ipaddress() module and using your own custom regex. We’ll also have a look at setting up your own custom function.
Validating an IP address using the ipaddress() module
This is by far the simplest method and one of the most secure. The ipaddress() function won’t actually tell you that an IP address is valid as such, but it can perform basic arithmetic with it. With a bit of custom juggling, we can use this to return a result about its validity.
The first step is to import the ipaddress() module into your code. Then, call the .ip_address() method on the ipaddress class, and pass it an IP string. If it’s a valid IPv4 or IPv6 address, the ipaddress module will use the string to produce a new IP address object.
If, on the other hand, the string you pass it is not a valid IP address, the ipaddress() function will return a value error. As we already explained, the module tries to use the string you provide to create a Python IP address object. So in order to actually validate an IP, you have to create your own IP validation function. Here’s an example:
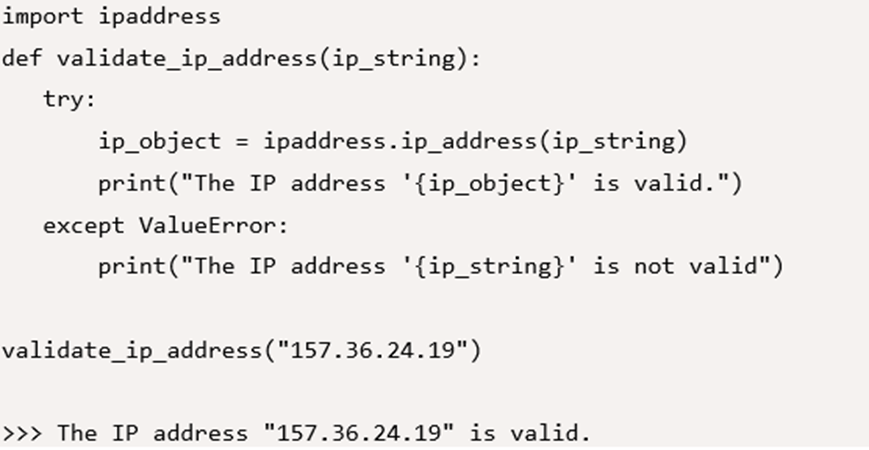
Note: this will also work with IPv6 addresses.
Validating an IP address with a custom regex
If you’re used to working with Python frameworks, you’ll appreciate its versatility as a coding language. So there are multiple approaches possible for any given coding task. Another way we can determine whether an IP address is valid or not is by using a regular expression (regex) to match the required pattern.
In this case, that means four numbers between 0 and 255 separated by dots. Because the values of the numbers are restricted to 0 ≤ n ≤ 255, you’ll have to add some more code of your own for additional verification.
(Also, since you’ll be defining the shape of the address, that means that you’ll need to write separate functions to check IPv4 and IPv6 addresses, since their shapes are very different.)
What you’re going to do is import the re library so you can use the .match() method to check whether your string matches the defined pattern.
After you’ve done this, the next step is to create your regex string. This defines the shape for matching. There are several ways of doing this, but here’s a straightforward example:
“[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}”
Let’s have a look at what’s going on there. This expression consists of:
[0-9] – you want numbers between 1 and 9
{1,3} – you want between 1 and 3 digits in each section
\. – tells the parser to look for the “.” symbol. This is important because in regex, “.” is a special character meaning “any character”, so if you want the parser to look for the character “.” itself, you have to include the backslash to tell it to do that.
Together, these components define one byte, so you require four of them to define the pattern of the IP address as a whole. (Although don’t forget not to put “\.” at the end – you only need to put them between each section.)
This is okay as far as it goes, but there’s more work to do. That’s because you haven’t yet specified that the value of each number has to be between 0 and 255. At the moment, a number like 495 would return a positive match. So you need to set up an additional check to verify this.
Putting it all together, you’ll end up with something like this:
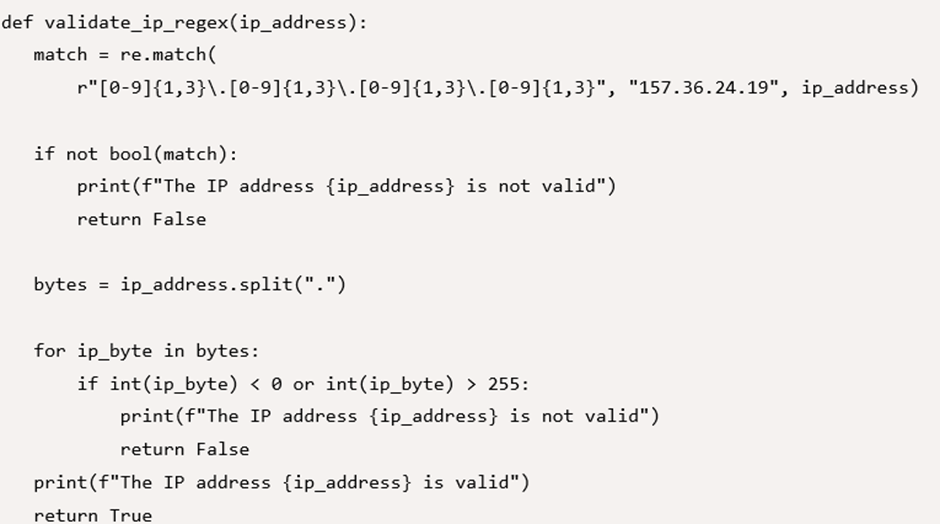
Validating an IP address using a custom function
Just as there are billions of IP addresses being used all over the world, whether on a desktop, a hosted IP phone, or a laptop, sometimes it feels as if there are billions of ways of achieving the result you want with Python.
So let’s look at one method of validating IP addresses in a slightly more freestyle way.
Breaking down the task into its constituent parts, we can see that we’ll need to do the following:
- Split up the IP address into four sections and store each section into a list of strings
- Verify that the IP string comprises four numbers separated by periods
- For each number in the IP string, verify that the number is an integer n where 0 ≤ n ≤ 255
We can use the len() function to determine string length. What you end up with might look something like this:
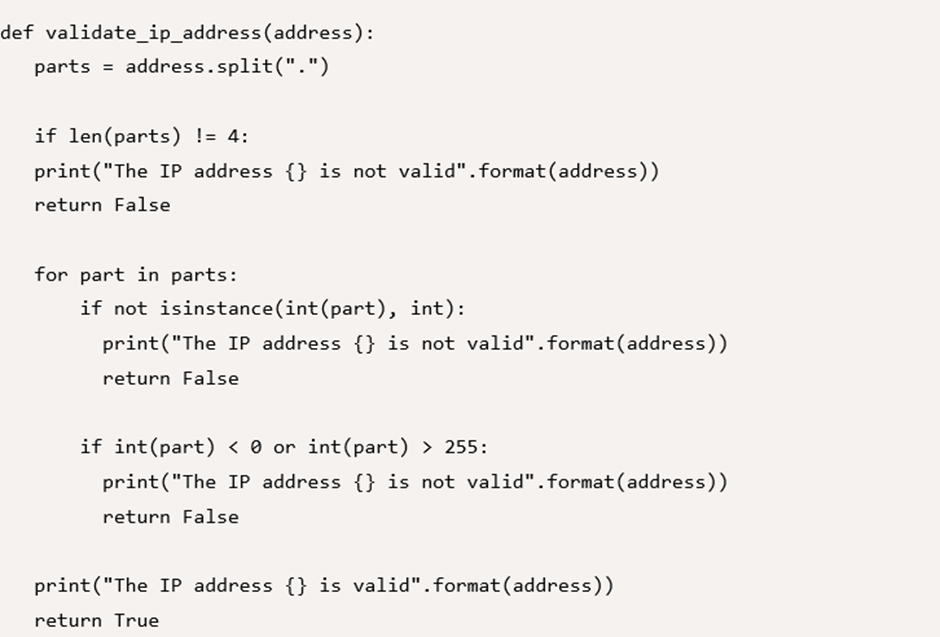
A note on Python
There’s a reason why Python is often listed as one of the essential programming languages for professionals. It’s a general-purpose language and one of the easiest to learn. However, its flexibility makes it a very powerful tool for meeting all kinds of programming goals.
As well as being equipped with many and varied features, the fact it’s open-source and so widely used means that there are plenty of resources available to learn how to use it. There’s a sizeable global community of developers very happy to share their expertise.
So whatever you’re working on, from design applications to projects aimed at mitigating VoIP security risks, there will always be a friendly expert ready to talk you through best practice.
You’ll come across Python in any number of contexts. It’s perhaps most commonly used for building websites and for development testing. But you’ll also see it in data science, prototyping and the creation of recommendation algorithms.
If you’re involved in writing software for self-driving cars or any number of projects managing VoIP for business clients, you may well also encounter Python in your day-to-day work. So it’s vital to get to grips with this increasingly important language.
Final thoughts
Remember that there are many different possible methods for validating an IP address using Python. We’ve covered three of the most common ones here, but you may very well prefer another.
That’s the beauty of coding, of course. Each developer has their own preferred style and way of achieving the same end goal. So feel free to experiment!