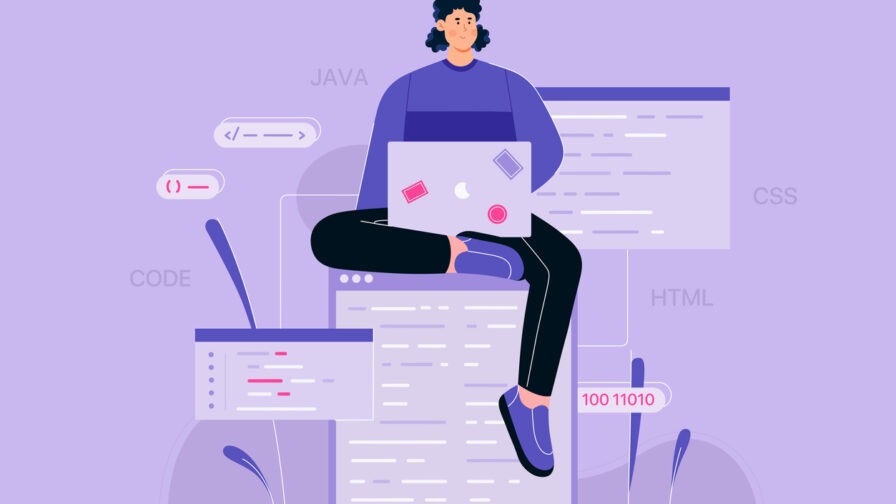
SASS comes with functions to programmatically modify colors, such as lighten()
and darken()
. These provide a practical approach to color manipulation in CSS. But what if you wanted to achieve the same result in plain JavaScript?
Considering how popular styling HTML components in JavaScript has become, that is a now basic need. As a result, several JavaScript color library options have sprung up. Their goal is to make it easier to deal with colors in JS. Here, you will dig into color
, the most popular one!
In this article, you will find out why you need a color JavaScript library, why color
is the best one, and what features and methods it offers.
Let’s dive in!
Why Manipulate Colors in JavaScript
Colors play a vital role in web design, user interface, accessibility, user experience, and data visualization. With the proper JavaScript color library, you can manipulate colors dynamically and programmatically in the frontend or backend.
In detail, there are at least three real-world scenarios why dealing with colors in JavaScript is important:
- Set colors in JavaScript code: Suppose that an API returns styling information about a specific component, or that you need to change colors based on user interaction. In this case, you cannot rely on CSS. Instead, you must enforce the styling of HTML elements in the frontend via JavaScript code. For example, in React this comes down to programmatically setting the
style
prop. - Convert colors: HEX, RGB, RGBA, HLS, and HLSA are the main formats that web applications support for color representation. The best format to adopt changes from use case to use case. Thus, you may need to convert colors to make them easier to understand or manipulate.
- Enhance accessibility: Give users the ability to select a specific theme and have all colors update accordingly. This is a great way to make applications and websites highly accessible.
Now, the question is, “Is there a library for all this in JavaScript?” Yes, there is, and it is called color
!
What Is the JavaScript color
Library?
color
is a JavaScript library for converting and manipulating colors. The npm package has more than 15 million weekly downloads, making it the most popular color manipulation library among all available alternatives.
color
exposes a Color()
constructor that accepts a color in several formats:
// CSS color strings
const color = Color("red")
const color = Color("rgb(255, 0, 0)")
const color = Color("#FF0000")
const color = Color("hsl(0, 100%, 50%)")
// object
const color = Color({r: 255, g: 0, b: 0})
Code language: JavaScript (javascript)
Behind the scene, string constructors are handled with the color-string
package.
The object returned by Color()
exposes several color conversion and manipulation methods. These are immutable as they always return a new color object, without changing the original data structure.
Using color
in JavaScript
Let us now look at the features offered by color
to implement color manipulation operations effortlessly.
Get Started
Install the color
npm package with:
npm install color
If you are a TypeScript user, you will also need to install the type declaration with:
npm install @types/color
Code language: CSS (css)
Then, you can import color
in Node.js with:
const Color = require("color")
Code language: JavaScript (javascript)
Or, as an ES module with:
import Color from "color"
Code language: JavaScript (javascript)
As mentioned before, Color
is a constructor function that accepts a color representation in many formats and returns an object that exposes several useful methods. Time to see them in action!
RGB to HEX and HEX to RGB
You can convert a color from RGB to HEX in JavaScript with color
as in the example below:
const blueRGB = "rgb(0, 0, 255)"
const blue = Color(blueRGB)
const blueHEX = blue.hex().toString()
console.log(blueHEX) // "#0000FF"
Code language: JavaScript (javascript)
The hex()
method returns a color object with data stored in HEX format. In particular, if you log blue.hex()
with console.log()
, you will see:
{ model: 'rgb', color: [ 0, 0, 255 ], valpha: 1 }
Code language: CSS (css)
Then, toString()
transforms that object into a human-readable CSS color string.
Similarly, you can go from HEX to RGB in JavaScript with:
const blueHEX = "#0000FF"
const blue = Color(blueHEX)
const blueRGB = blue.rgb().toString()
console.log(blueRGB) // "rgb(0, 0, 255)"
Code language: JavaScript (javascript)
Here, you need to rely on the rgb()
method instead.
Convert a Color to a Different Space
color
offers several methods to convert from one color space to another. As of this writing, these space color conversation methods are:
rgb()
: To convert a color from any supported color space to RGB.hsl()
: To convert a color from any supported color space to HSL.hsv()
: To convert a color from any supported color space to HSV.hwb()
: To convert a color from any supported color space to HWB.cmyk()
: To convert a color from any supported color space to CMYK.xyz()
: To convert a color from any supported color space to CIE XYZ.lab()
: To convert a color from any supported color space to L*a*b.lch()
: To convert a color from any supported color space to LCH.ansi16()
: To convert a color from any supported color space to 16 ANSI.ansi256()
: To convert a color from any supported color space to 256 ANSI.hcg()
: To convert a color from any supported color space to HCG.apple()
: To convert a color from any supported color space to the Apple color space.
Check If a Color Is Light or Dark
To evaluate whether a color is light or dark, you can simply use the isLight()
method:
const lightBlue = Color("lightblue")
console.log(lightBlue.isLight()) // true
Code language: JavaScript (javascript)
Or the opposite isDark()
method:
const darkBlue = Color("darkblue")
console.log(darkBlue.isDark()) // true
Code language: JavaScript (javascript)
Note that if isLight()
returns true
, isDark()
will return false
and vice versa:
const lightBlue = Color("lightblue")
console.log(lightBlue.isLight()) // true
console.log(lightBlue.isDark()) // false
const darkBlue = Color("darkblue")
console.log(darkBlue.isLight()) // false
console.log(darkBlue.isDark()) // true
Code language: JavaScript (javascript)
Under the hood, these two methods rely on the YIQ equation for calculating color contrast.
Manipulate Colors
Other useful methods to manipulate colors offered by color
are:
lighten()
: To lighten a color by a specified percentage.
blue.lighten(0.3) // #4D4DFF
Code language: JavaScript (javascript)
darken()
: To darken up a color by a specified percentage.
blue.darken(0.2) // #0000CC
Code language: JavaScript (javascript)
lightness()
: To Set the lightness of a color to a specific value.
blue.lightness(35) // #0000B3
Code language: JavaScript (javascript)
saturate()
: To saturate a color by a specified percentage.
blue.saturate(1) // #0000FF
Code language: JavaScript (javascript)
desaturate()
: To desaturate a color by a specified percentage.
blue.desaturate(0.5) // #4040BF
Code language: JavaScript (javascript)
whiten()
: To whiten a color by a specified percentage.
lightBlue.whiten(0.1) // #BEDCE6
Code language: JavaScript (javascript)
blacken()
: To darken a color by a specified percentage.
lightBlue.blacken(1) // #ADC5CD
Code language: JavaScript (javascript)
rotate()
: To apply a hue rotation on a color by a specified degree.
blue.rotate(180) // #FFFF00
Code language: JavaScript (javascript)
fade()
: To fade a color by a specified percentage
lightBlue.fade(0.2) // #ADD8E6
Code language: JavaScript (javascript)
opaquer()
: To make a color more or less opaque based on the specified percentage.
lightBlue.opaquer(0.8) // #ADD8E6
Code language: JavaScript (javascript)
grayscale()
: To get the grayscale equivalent of the current color.
blue.grayscale() // #1C1C1C
Code language: JavaScript (javascript)
Paste the resulting color in the JavaScript comment in a HEX color visualizer to see the effects of each method.
Other Getters
color
also provides many getter methods to get specific information about a color:
red()
: To get the “red” component of the color in the RGB space.green()
: To get the “green” component of the color in the RGB space.blue()
: To get the “blue” component of the color in the RGB space.alpha()
: To get the “alpha” component of the color in the RGBA space.lightness()
: To get the lightness of the color according to the HSL space.keyword()
: To get the CSS name of the color.cyan()
: To get the “cyan” component of the color in the CMY space.magenta()
: To get the “magenta” component of the color in the CMY space.yellow()
: To get the “yellow” component of the color in the CMY space.black()
: To get the “black” component of the color in the CMYK space.x()
: To get the “x” component of the color in the CIE XYZ space.y()
: To get the “y” component of the color in the CIE XYZ space.z()
: To get the “z” component of the color in the CIE XYZ space.l()
: To get the “L” component of the color in the L*a*b space.a()
: To get the “a” component of the color in the L*a*b space.b()
: To get the “b” component of the color in the L*a*b space.
With all these methods, dealing with color manipulation in JavaScript has never been easier!
Conclusion
In this article, you looked at how having the ability to deal with colors directly in JavaScript is so important. As seen here, the JavaScript color
library comes equipped with everything you need to perform color manipulation and conversion.
Through several examples, you had the opportunity to understand how to use color
in real-world scenarios. Converting from HEX to RGB and vice versa is now a piece of cake!
Thanks for reading! We hope you found this article helpful!