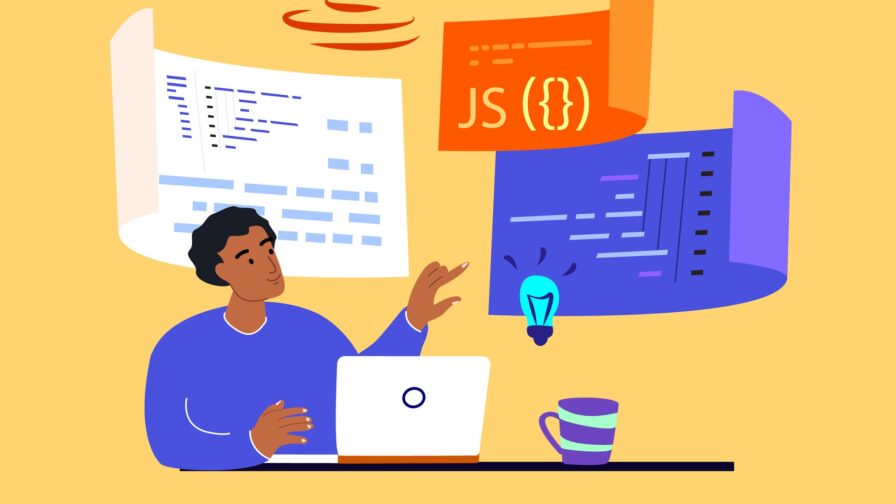
Data structures are formats in JavaScript that allow developers to organize, store, and manage data in a variety of ways.
A variety of different data structures are available for use, which can be used to solve a whole range of real-world problems.
We’re going to take a look at the ins and outs of some of the most commonly used JavaScript data structures, what benefits they can provide to developers, and some of their most common use cases.
What is a JavaScript Data Structure?
A data structure in JavaScript is a way of organizing and storing data that allows developers to efficiently perform operations on that data.
They are formats that can be applied to data which allow more efficient access to the data and allow modifications to be made to it when required.
JavaScript provides several built-in data structures, which provide fundamental tools for developers to manage and manipulate data in programming.
Understanding these different data structures, and their specific uses, can help developers to choose the right structure for their requirements.
What are the Benefits of Using JavaScript Data Structures for Developers?
Using JavaScript data structures offers several benefits for developers, often helping to make their programming tasks, such as streaming data, more efficient and streamlined.
Because JavaScript provides built-in data structures like arrays and objects, developers can quickly create and manipulate data without the need for external libraries or complex setups.
Data structures like arrays, sets, and maps are designed to handle data incredibly efficiently and offer fast access, insertion, and deletion operations. This enables developers to optimize the performance of their applications, and greatly streamline their processes and programming tasks.
JavaScript data structures are also designed to use memory efficiently, which can be crucial when working with large datasets, or optimizing the performance of memory-intensive applications. This makes them a useful MLOps solution.
Not only that but choosing the right data structures can improve code readability and maintainability. For example, using a map to represent a key-value relationship instead of nested arrays can make the code more intuitive and easier to understand.
The flexibility provided by JavaScript data structures allows developers to work with diverse data sets, and solve many different types of problems. JavaScript data structures can store a wide variety of data types, including numbers, strings, objects, and even other data structures.
Many of the algorithms and problem-solving techniques used by developers rely on specific data structures. Because JavaScript features built-in data structures, it is easier for developers to implement these algorithms, and efficiently perform data manipulation tasks.
Due to JavaScript’s popularity, there is a vast community of developers using these data structures who are sharing knowledge, libraries, and resources related to them. This support makes it easier for developers to learn and leverage the full potential of data structures.
7 JavaScript Data Structures Developers Use to Solve Real-World Problems
The wide range of data structures available in JavaScript allows developers to perform a range of different functions, whether it’s building an ETL framework, carrying out data analysis and visualization, or creating AI and ML algorithms.
Stack
A stack is a linear data structure that holds a list of elements. It works on the principle of LIFO (Last In, First Out). This means the most recently added element to the stack is the first to be removed.
There are two main operations that occur at the top of the stack; push and pop. With the push method, you can add one or more elements to the end of the array. With the pop method, you can remove the topmost element at the end of the array, which is then returned to the caller.
Stacks are not natively available in JavaScript, but they can be added using a relatively simple array:
const stack = [];
// add item to the "top"
stack.push("value");
// remove item from the "top"
const topItem = stack.pop();
Code language: JavaScript (javascript)
A stack can be used in the real world when you need to keep track of the history of user interactions. This can be used to build functions such as an ‘undo’ button.
Queue
Queues are similar to stacks in some ways, with one key difference. Rather than removing the element that was added last, a queue instead removes the element that was first added to the queue. In this way, queues operate on a ‘First In, First Out’ (FIFO) principle.
With stacks, queues are not natively available in JavaScript, and so must be added using an array:
const queueu = [];
// add item to the "end"
queueu.push("value");
// remove item from the "beginning"
const firstItem = queueu.shift();
Code language: JavaScript (javascript)
A queue can be used in the real world to display notifications on top of each other. After a while, the oldest notifications will disappear from the screen, making room for more recent notifications to take their place.
They have applications in software features such as the call waiting features of PBX cloud services.
Queues can also be used in batch processing to ensure that data is analyzed in the correct order, or in task scheduling where they can help to schedule tasks in the order they were received.
Priority Queue
A priority queue operates similarly to a standard queue but with the ability to allocate priority to each element. This allows for elements to be sorted in order of their priority.
The element with the highest priority will always appear at the front of the queue, and will be replaced by the element with the next highest priority if it is removed.
Priority queues have applications in event-driven simulations such as network simulations, enabling developers to determine the correct order of events in which to process events.
They also have applications in medical environments, for example in the triage systems of emergency departments. Here they can be used to prioritize patients based on the urgency of their condition, allowing the most critically ill patients to be seen first.
Set
In JavaScript, a set is a group of definite, distinct objects. Each item in a set must be unique, meaning that no elements are repeated.
A set can be created in JavaScript using the following code:
let arr = [1,2,3,4,4];
const set = new Set(arr)
Code language: JavaScript (javascript)
Sets have various real-world applications, including deleting duplicate elements from an array. Converting a list into a set in JavaScript will automatically eliminate any duplicate elements.
Sets are also efficient at checking if a specific element exists within a collection, which can save a lot of time for developers who need to scour large datasets for specific elements when carrying out big data visualization.
Linked List
A linked list is a type of chained data structure in JavaScript. It connects various elements, known as ‘nodes’, through links.
Each node consists of two key features; the data it contains, and a reference, or ‘pointer’, to the next node in the sequence.
Linked lists are commonly used in scenarios where dynamic data insertion or deletion would be beneficial. Because the elements of lists are not stored in contiguous memory, but instead scattered in memory and connected by pointers, linked lists allow for dynamic memory allocation, enabling flexible deletion and insertion operations.
Real-world examples of the use of linked lists include web pages which can be navigated using ‘previous’ and ‘next’ URL links. For example, tutorials on how to access iPad remotely could use linked lists for easy navigation through the steps.
Graph
A graph in JavaScript is similar to a linked list in that it uses a series of nodes, or vertices, connected via paths, or edges.
Graphs can be further divided into two groups; directed and undirected graphs, based on whether the paths have an associated direction.
In the real-world, graphs have applications such as helping to calculate the best route in a navigation app, or recommending friends to potentially connect with on social networking sites like Facebook.
They are also instrumental in optimizing the layout of an online store, making them invaluable for a Shopify theme developer.
Tree
A tree is a type of nested data structure within JavaScript. They are hierarchical structures containing a single root node, known as a ‘parent’.
This parent has ‘children’, which are elements nested within the original root node. Each of these children may have further children nested within them.
Trees can be defined in various ways. One method is to use a flat array, which requires each element to possess an ID, and a reference to their parent and any associated children:
[
{
id: "parent-1",
name: "Parent",
// references to children via their IDs
children: ["child-1", "child-2"]
},
{
id: "child-1",
// reference to the parent vid its ID
name: "Child 1",
parent: "parent-1"
},
{
id: "child-2",
name: "Child 2",
parent: "parent-1",
children: ["grandchild-2"]
},
{
id: "grandchild-21",
name: "Grandchild 21",
parent: "child-2"
}
]
Code language: PHP (php)
A tree can be used to set up nested menus on a website or to create a hierarchical structure for organizing client accounts and services in a web hosting control panel.
Use JavaScript Data Structures to Solve All Manner of Real-World Problems
JavaScript data structures have a multitude of different uses, allowing developers to tackle a whole range of different real-world problems involving data analysis, web development, and even AI!
Whether it’s using a stack to track user interactions, or building a nested comments section using a tree, there’s a JavaScript data structure for every job.