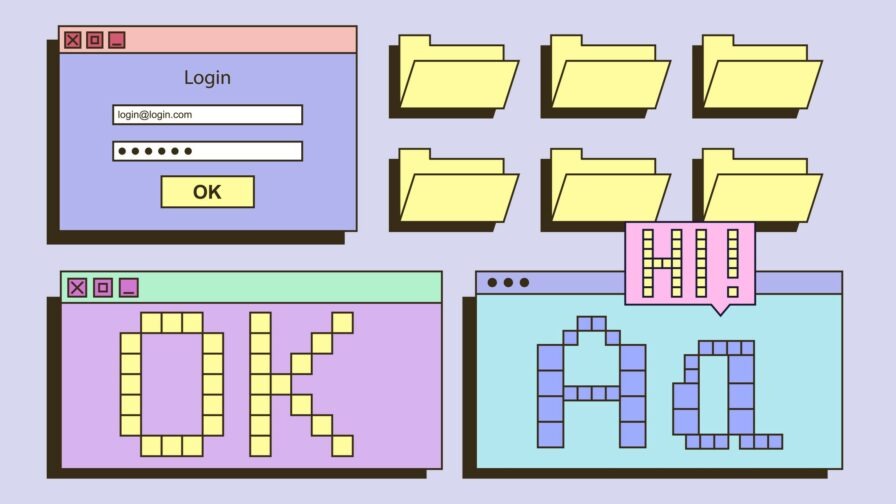
When building web applications, security is a key part of the development process. A super-cool app might come with a fantastic UI, but if it is highly vulnerable to attack by malicious actors, that’s a problem – especially if the app handles sensitive data or banking transactions.
Application security management involves many operations, from back-end checks to route protection, different authentication and authorization processes, and the construction of secure queries to the database. However, the first layer of protection in an application involves validating user inputs. This fundamental task is managed by the back end, but the front end is also involved, with the goal of increasing the application’s usability quality.
This article looks at how to enforce front-end validation, improving the user experience through five simple, but powerful, rules.
Security: front end vs back end
Validation is a key part of the security process. Validation allows the app to determine if the inputs typed by the user and the operations they are performing are correct and what the application expects. The golden rule is that real validation only takes place in the back end, so why discuss front-end validation? Because front-end validation is immensely useful for increasing the quality of the user experience, which is a determining factor in keeping users on the app by offering them a satisfying experience.
Imagine having to fill in a very long form, consisting of inputs, radio buttons, drop-down menus, checkboxes, then sending everything to the back end, receiving an error message relating to one or two fields, and having to recompile everything. It’s a pretty painful experience for the user. Sure, the problem can be buffered with input repopulation back-end operations, but wouldn’t it be better to prevent bad data from being sent in the first place? This is where front-end validation comes into play.
It’s important to remember that everything on the front end can easily be deactivated or bypassed, so its function lies mainly in UX and resource-saving. The back end will always have to check the incoming data as well as taking care of many other security tasks.
On the front end, the goals are:
- increase in success rates
- increase in satisfaction rating w
- decrease in errors made
- decrease in completion times
- decrease in the number of eye fixations
Front-end validation production mainly goes through JavaScript (in combination with HTML and CSS); whether using a framework or library such as React or Vue, or writing Vanilla code, this remains the same.
Here are five general rules to apply for great front-end validation, whatever the development stack.
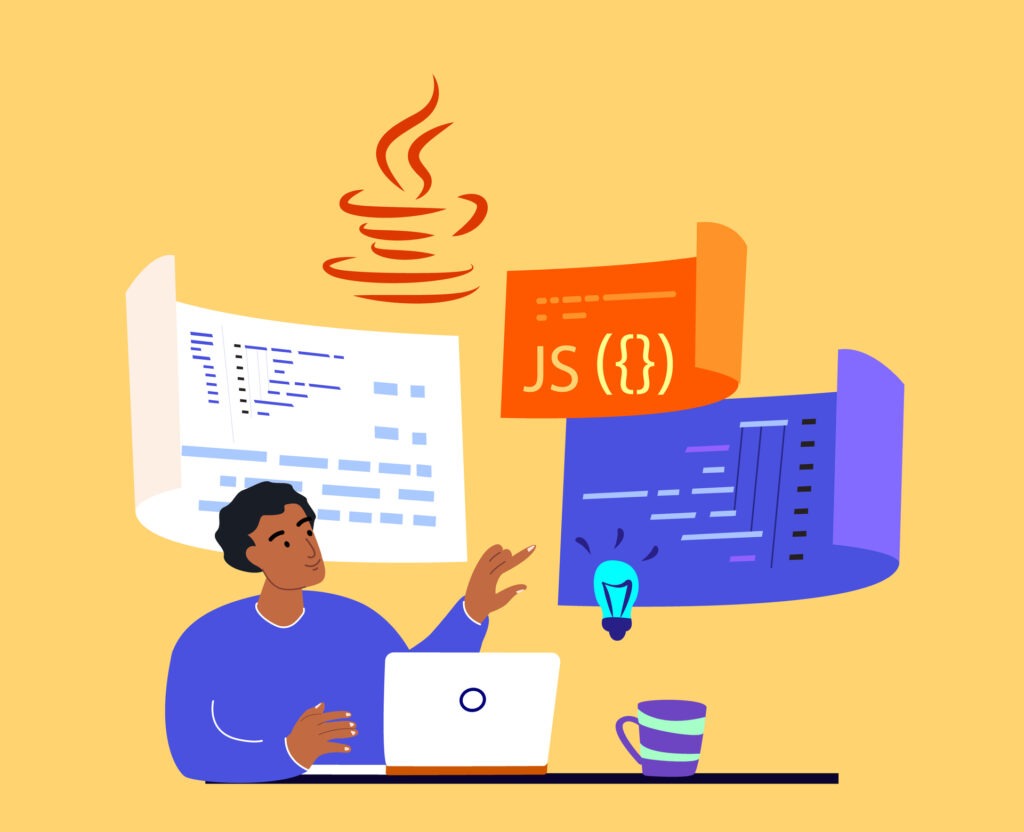
The golden rules of UI and Security
Rule #1: the input should be what the back end expects
As previously stated, the goal of the front-end validation is to ‘clean’ the data sent to the back end, ensuring it is in the correct format, shape and range. The back end will perform all the necessary checks, but the production of error messages on the front end will be drastically reduced. For example, if a select field sets a choice between the colors blue, red, and green, but the user selects or types the yellow value, the front-end algorithm must verify that the value selected is out of range, and report this to the user before uselessly sending the entered data to the back end.
This procedure must occur for all fields, paying attention to:
– empty inputs or those that contain unsuitable values (special characters, etc.)
– string and numerical values outside the desired range
– incorrect patterns (date, time, passwords, e-mail, etc.)
– missing required data
This ‘filtering’ is useful for both security and user experience.
There is also another very useful procedure for sending correct data to the back end: checking (usually via AJAX request) for data that should not already be present, such as nicknames and e-mail addresses. When the user enters a username or an e-mail, the front-end algorithm has to both verify that these have not already been selected by another user, and asynchronously show the error so that the user can select other values before sending them all to the server.
However, because the user can disable JavaScript, model the DOM on-the-fly, or manipulate the sending of headers and payloads to the server, these checks are not conclusive. As a general rule, this can be summarised as: never trust user-input data – a cliché any code-writer has probably heard once or twice!
Rule #2: make regular expressions a good friend
Regular expressions are a fundamental component in any validation and security procedure. While they can be a complex subject, being good with regular expressions is nonetheless of paramount importance. There are many ‘prepackaged’ regular expressions, such as validating a correct date format, that a secure password matches specific criteria, or that a valid username has been entered, but since each application is different, these may need to be customised to best suit the app’s needs.
The positive note is that although the syntax varies between language and language, the central basis of regular expressions is universal. Here too, the regular expressions used on the front end have the aim of improving the UX and relieving the production of errors, so the same checks must also be applied on the server side.
Mastering and using regular expressions allows the performance of advanced validations, thereby assisting in putting rule # 1 into practice even in situations that require more complexity.
Rule #3: dynamic error reporting, but success messages too
As discussed already, reporting errors in real-time is of great importance to allow the user to fix mistakes before sending data to the back end. However, the presence of success messages is also very important; these allow the user to understand without a shadow of a doubt that what they are compiling is correct.
Knowing that the username typed in is correct is just as important as knowing that it isn’t. Similarly, knowing why the password the user is entering is not correct is useful for correcting it; here the presence of regular expressions is useful for reporting precise errors (the lack of a number, a capital letter, etc.) allowing users to check that the entered value reflects all the criteria set by the application.
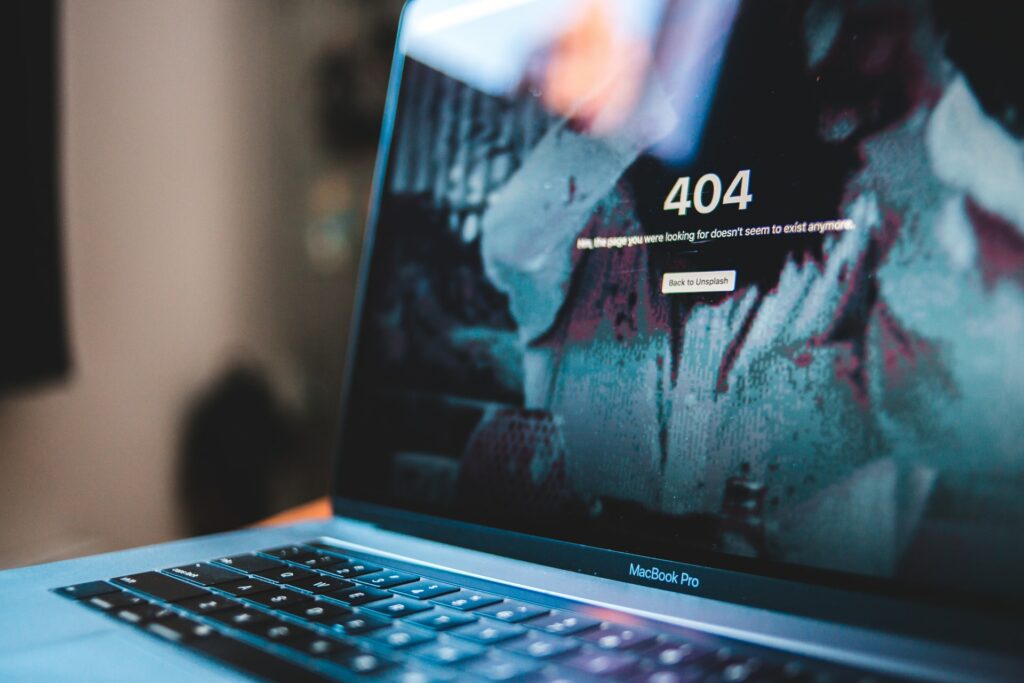
Where to show such errors, and in what format, is a front-end specific task. The most commonly accepted practices require the presence of error or success messages next to or below the inputs, using a colour palette that aligns with the application’s UI.
As a universal rule, never rely on colours alone to communicate error or success data. For people with red-green colour blindness, green and red look almost the same, so it;s likely to be difficult for them to know if an error or success has occurred. A better approach would be to add an icon to make this unequivocally clear.
Rule #4: if the input is incorrect, prevent form submission
Supporting rule # 1 further, the problem of sending incorrect data to the server can be rooted out by simply preventing data from being sent at all if any field fails validation. By using JavaScript to keep dynamic track of the fields that pass the relevant validation and those that do not, submission of the form can be activated or deactivated.
This process also makes it obvious when the form has problems: the submit form can either be prevented from appearing, or functionally and graphically disabled via CSS.
By writing consistent and good quality code, the addition or removal of new input fields of different types in the page structure is simple, as is a painless integration of additional controls into the JavaScript algorithm/component.
Bonus Rule: what if Javascript was disabled?
All the rules listed previously have had a common need: JavaScript must be enabled in the browser. However, JavaScript can easily be disabled, either for security reasons or to try to bypass certain security measures.
Therefore, take into account that rule #1 must stay as active as possible even when JavaScript is disabled. In this case, the power of HTML 5 and CSS can be of real help, allowing the setting of ranges and the prevention of invalid input being typed and sent. For example, these can be used to build SEO-friendly semantic inputs like the ‘email’ type to check against a valid email pattern, a ‘required’ attribute to mark a field as mandatory, or the less commonly known ‘pattern’ attribute to perform a match against a regular expression pattern:
<label for="color">What is your favorite color? (required)</label>
<input required pattern="[a-zA-Z]{3,20}" type="text" id="color" name="color">
CSS also facilitates the creation of dynamic and reactive animations that are activated when certain events occur, and uses pseudo-classes such as “:required”, “:valid” and “:invalid” to set appropriate styles.
Although it’s obviously not possible to replace the presence of an enabled Javascript completely, it is possible to prevent the insertion of incorrect data, in accordance with the objectives of rule #1.