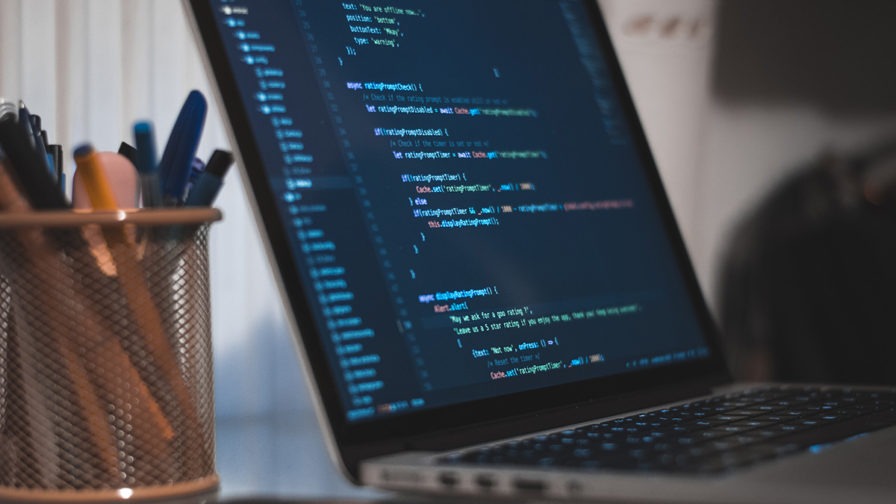
- You are already know TypeScript (almost)
- It is very easy to get started with TypeScript
- Compilation has its adavantages
- Data binding is safer in TypeScript
- TypeScript allows OOP like a pro
- Reuse code with type-safe generics
- TypeScript has decorators that don’t decorate
- Conclusions
- Want to launch a developer event despite the challenges of COVID-19?
One of the most intriguing innovations of web technologies is the TypeScript programming language. Although very powerful and easily integrated into the existing JavaScript ecosystem, it is not as widely adopted yet.
Nevertheless, it has the potential to change dramatically the way we design web applications. It is no surprise that this is one of the hottest topic at the Spanish edition of Codemotion Online Tech Conference, which will be held on November 3-4-5. If you’ll attend (tickets are free), you should not miss the Manuel Carrasco Moñino’s talk about The perfect cocktail: Java + Typescript (check the agenda here).
If you have been away from web development in the last years, coming back to it could be quite a shocking experience. Basically everything you knew has changed. Things are evolving so fast that it feels like it keeps on changing literally as you type code.
Even good old JavaScript is almost unrecognizable under all that heavy framework make-up, and now it runs server-side too! The good news is that even if you have to learn new stuff, the change is for good and can help you writing less and better.
Learn TypeScript and you will unleash the power of strongly typed languages into the JavaScript ecosystem. But more important, you will have an expressive language to model complex applications.
You are already know TypeScript (almost)
TypeScript is a super-set of the JavaScript programming language, so it is not very different from what you are used to if you are familiar with web development.
Though it has several distinctive features, TypeScript is designed to integrate seamlessly with the JavaScript ecosystem. This means that it is completely transparent for the target environment, giving you the freedom to use TypeScript everywhere you can run JavaScript.
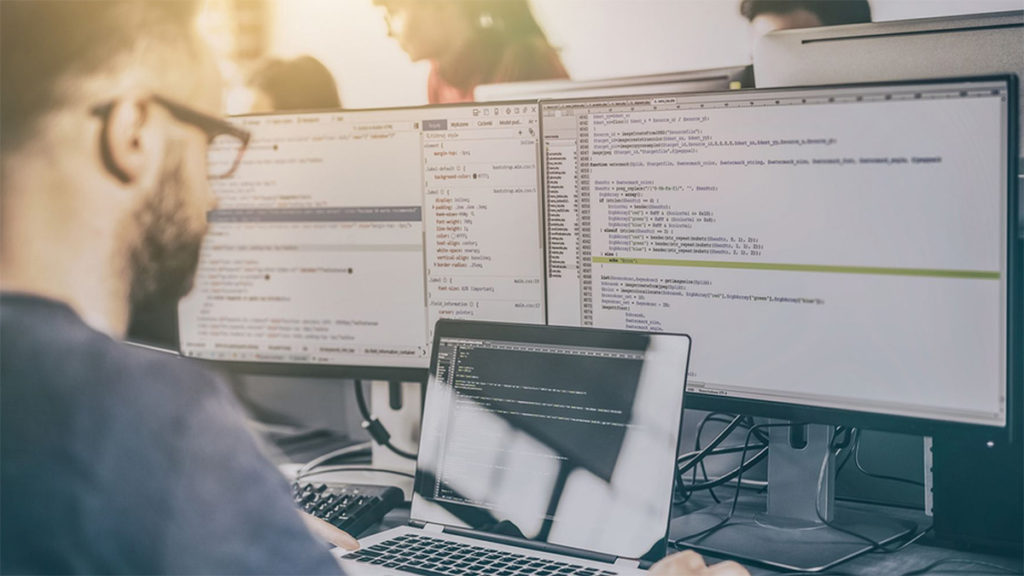
It is very easy to get started with TypeScript
Getting started with TypeScript is quite simple, especially if you are used to Node.js. The following command will install the TypeScript tools on your computer:
npm install -g typescript
Now you can start experimenting with your IDE of choice. Many tools already provide an extended support to TypeScript features. As an example, Visual Studio Code has built-in syntax-highlight, code completion and debugging utilities that will make TypeScript programming a smooth experience.
Compilation has its adavantages
One of the major breakthrough of TypeScript over JavaScript is the fact that TypeScript is a compiled language.
Technically speaking, compiling TypeScript will not produce machine code. It is indeed a translation to plain JavaScript, therefore the verb transpile is often used to refer to this step.
As for other languages, the use of a compiler is an enabling factor for the implementation of static syntax checking. For the compilation process to be successful, every part of your program must comply with TypeScript syntax rules, while when you interpret JavaScript code, the checks are done only at run-time. This means that only the parts of your code that are effectively executed will be analyzed by the interpreter and therefore, any modification to your JavaScript program has the potential to introduce regressions, silently breaking even untouched lines of your code.
TypeScript code is compiled to plain JavaScript with the tsc command line tool:
tsc hello.ts
Code language: CSS (css)
As result of the compilation step, the output file hello.js will be created, ready to be interpreted by the browser or, if you have the Node run-time installed on your computer, with the following command:
node hello.js
Code language: CSS (css)
Data binding is safer in TypeScript
Types, as the name itself implies, are the core feature of TypeScript. Since JavaScript is a loosely typed language, data binding is a challenging task when developing complex applications.
The use of types in object definition allows to check at compile time if the data structure you are using fit in the code that is handling it. As an example, a number can be used in arithmetical operations, while a string is not suitable. The following code snippet show the use of types in variable definition, function arguments and return values:
Compiling this code will issue an error message to let you know that a string is not supposed to be used in a context designed to handle only numeric data in TypeScript. Nonetheless, the compilation process will output well-formed JavaScript code that will print on screen a NaN value (not a number) when executed.
While in this trivial example it is not a big deal whether you print a valid number or not, in other contexts it could be critical. Therefore, being notified of this potential issue at compile time will allow you to fix this behavior in time.
Types however, are optional in TypeScript since it is totally back-compatible with JavaScript. This explains why these errors are not critical for the compilation process.
TypeScript allows OOP like a pro
Class definition and the inheritance model in TypeScript mimics all the major syntactic features of high-level OOP programming languages like Java or C#, though it is based on the prototyping chain system of JavaScript.
The major advantage is the capability of outlining complex data models with a compact syntax that matches the expectations of developers that are familiar with OOP.
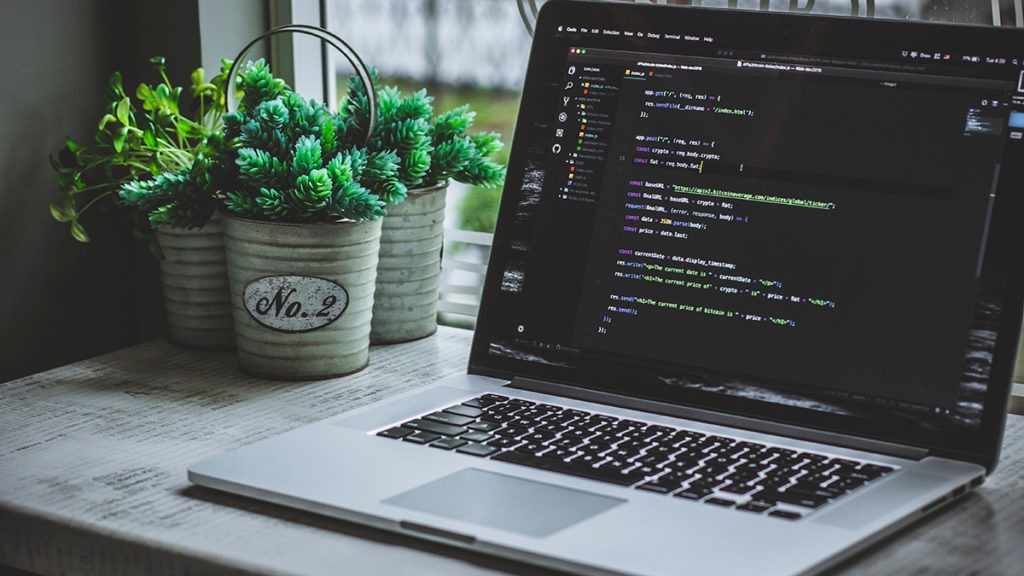
TypeScript delivers true encapsulation with access modifiers, polymorphic behavior with abstract classes and multiple inheritance through interfaces. This expressive power is unmatched even in the latest revision of the ECMAScript language, though it includes specific keywords for class definition and inheritance.
In a world where components have become the building blocks for modern web applications, this aspect of TypeScript is clearly appealing, as inheritance is one of the best ways to ensure code consistency and re-usability.
You may indeed think of web components as a way to reconcile OOP concepts with web development practices, and in this sense TypeScript comes ready to party.
Reuse code with type-safe generics
Compilation is also the enabling factor for the implementation of generics in TypeScript. Similarly to other high level languages, like C++ or Java, generic programming is a way of adapting the same piece of code to multiple data types.
Interfaces, similarly to classes, are used to define archetypal data types, but with an higher level of abstraction.
Generics and interfaces can be used together to implement type-safe template code, as shown in the following example:
In this example, the Validable interface restricts the use of the generic function validate only to instances that effectively define a method named isValid, regardless of the validation logic, that is very different for the Point and the Vehicle classes.
At the same time, the invocation of validate on objects that are not Validable will issue a compilation error. Once again, compilation prevents run-time errors on unsuitable instances and enables effective code reuse through generics.
Generics are one of those advanced features of TypeScript that you may not find needful at first. Nevertheless they allow to implement with ease a lot of design patterns that can fit in many scenarios, so they are definitely worth to understand at least in broad terms.
TypeScript has decorators that don’t decorate
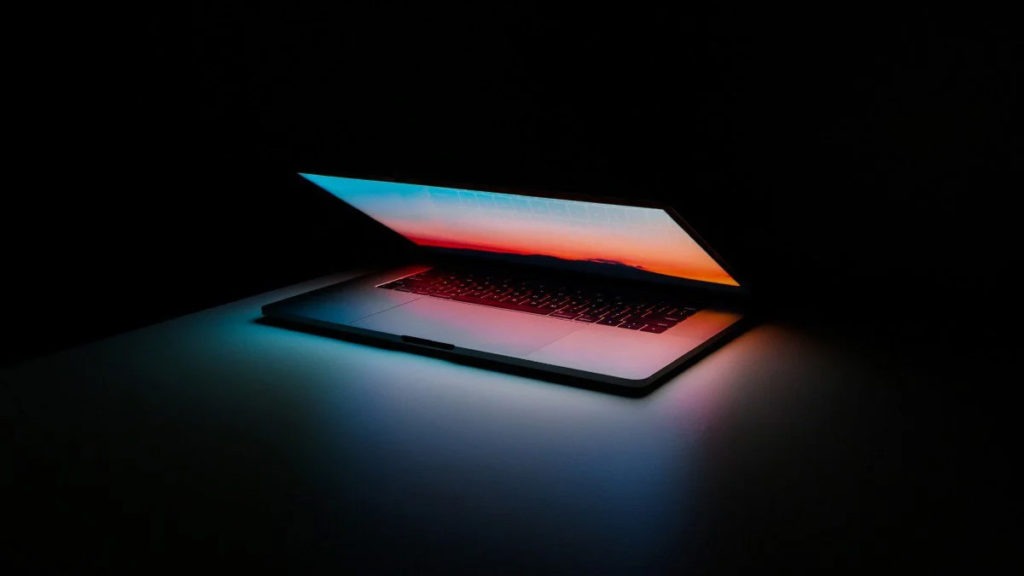
Decorators are still an experimental feature of TypeScript. You have to enable the support to compilation with the following options:
tsc source.ts --target ES5 –experimentalDecorators
Code language: CSS (css)
Decorators are applicable to classes, methods, properties and function arguments. Their name may sound a little misleading, since they are far from cosmetic, and actually apply deep changes to your code, in a meta-programming fashion.
For instance decorators are widely used in Angular for the sake of dependency injection. For the majority of developers, their use may fit in scenarios that require to address so-called cross-cutting concerns.
As the name implies, there are general requirements that apply to many parts of your business logic. User authentication for instance is a prerequisite to operations that require some level or privilege. Therefore, you may need to verify the credentials in many independent contexts. In this sense, authentication is a cross-cutting concern.
In many cases, the only option may be to implement the authentication checks in a specific function and invoke it everywhere you need it. Beside its simplicity, the major drawback of this solution is the need to actually modify working pieces of code to full-fill a non-functional requirement.
You may recognize this scenario as the perfect fit for aspect-oriented programming (AOP) and that’s exactly what decorators are meant for.
The following example gives a taste of what decorators can do in such a scenario:
A decorator is used to add authentication to the methods of a class that implement an HTTP service.
The function named authorizedOnly is attached with the decorator syntax @func_name to the methods of the dummy class MyService that need user authentication.
The body of authorizedOnly performs user authentication calling the external function isUserLoggedIn and on negative result, it injects an unnamed function in place of the decorated method that was originally invoked, showing an error message. In this example, authentication will always fail, unless you toggle the value returned by isUserLoggedIn.
No matter how complex the real business logic is, thanks to decorators it will be added to every method with a simple tag, and mostly important without modifications to the original code.
Conclusions
TypeScript is more than just JavaScript with types. Adding a strongly-typed sub system to a programming language has several side effects, the most relevant being the compilation process.
TypeScript’s static type checking and its advanced features like generics and decorators will probably extend the life of the JavaScript foundation for many other years to come.
Nevertheless, transitioning from JavaScript to TypeScript requires a little effort to recognize the significant differences between the two beyond the apparent similarities. But if want to be ready for the next generation of frameworks and tools, getting used to TypeScript now is a must.
If you want to learn more about this topic, don’t miss Manuel Carrasco Moñino’s talk, The perfect cocktail: Java + Typescript, at the Spanish edition of Codemotion Online Tech Conference on November 3-4-5: free tickets are still available!
Want to launch a developer event despite the challenges of COVID-19?
If you want to know more about how modern technologies and tools can support you for – and during – the organisation of a virtual event, don’t miss this article showcasing the best tools we used to host our online conferences since the COVID-19 outbreak.