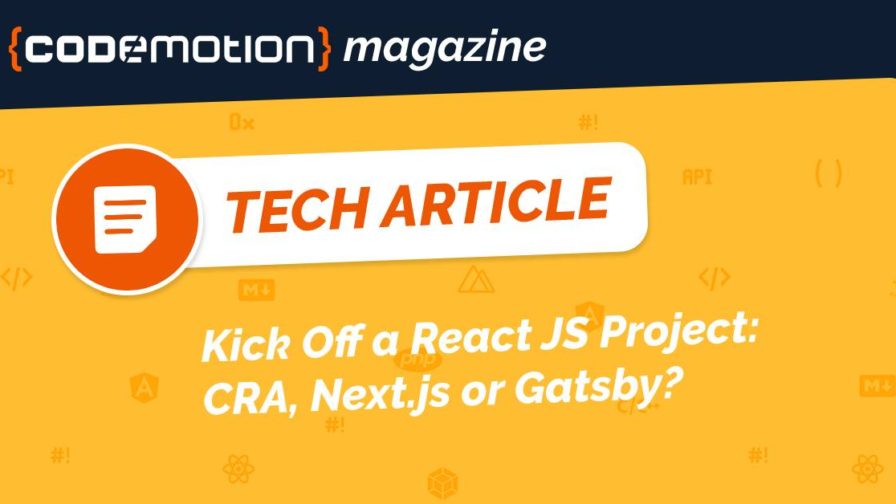
A simple guide to avoid getting lost in the React JS bootstrapping solutions. Learn more about CRA, Gatsby and Next.js.
This is a simple guide for normal humans to avoid getting lost in the React JS bootstrapping solutions out there.
React is a JavaScript framework designed to build a powerful single page app, developed on Facebook since 2013, which suggests a component-based approach to building applications.
The development of React JS is based on a declarative model, in which each component defines a portion of an app using the JSX syntax, and these components can be combined.
Many features have evolved from the original design of React code, such as the native JavaScript class support for React components. With this evolution, the number of features available to configure in a project pipeline increased, alongside the complexity of the configuration.
Starting a React JS project implies the configuration of multiple tools such as Webpack, Babel and so on, to create the foundations of your web app: depending on project requirements, the configuration can be complicated and hard to maintain.
This is the reason multiple code boilerplates started to pop up on code platforms like Github: the evolution of some of these boilerplates eventually led to the creation of more advanced and specialized tools such as CRA , Gatsby and Next.js to cater to common needs.
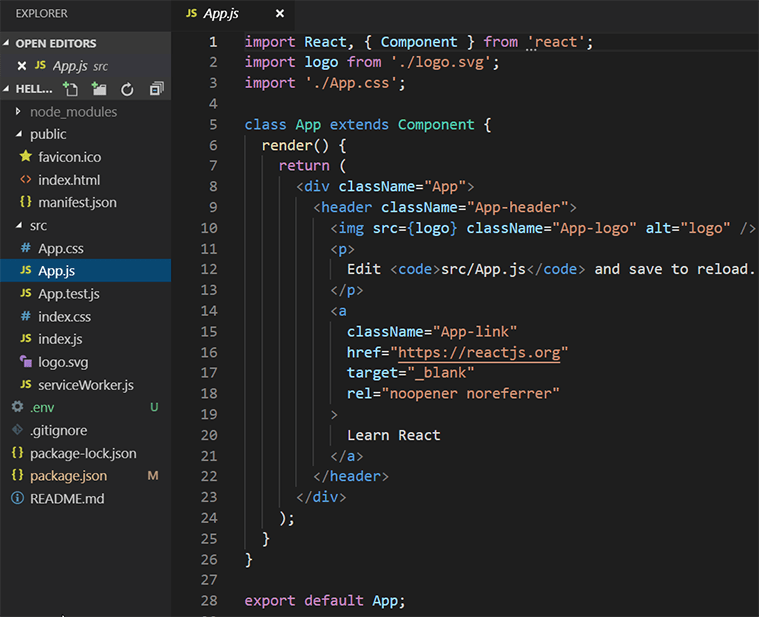
How to choose which new React JS project to build?
The first step in making a choice is to understand what problems the tool solves, and if these solutions match your needs. Let’s start from the end.
When to consider CRA
- The React app is single page and self contained on the frontend (no need for a server).
- The application relies on server APIs
- You want to experiment or get started with React
- You want to try a new React JS project and pick utility libraries on your own
When to consider Gatsby
- You are building a landing page
- You are building a static website (and the team likes ReactJS?)
- There are particular pages of your website in React that need to be super performers (move them to Gatsby if they are already in CRA for instance)
- You need better Search Engine Optimization (SEO) or AMP (Accelerated Mobile Pages)
When to consider Next.js
- The app is composed of both a server and a client side
- The React app is already in express.js and needs to be extended on the client side as well
- You are building a dynamic website
- You need SEO or AMP and you have dynamic content
CRA: create-react-app
Create-react-app (also known as CRA) is an official React CLI announced by the Facebook team behind ReactJS itself in 2016: the goal of the project is to provide a simple way to bootstrap your Single Page Application (SPA) in React.
A Single Page Application is a web app created out of a single HTML page that “virtually” involves many other pages created dynamically on the frontend side.
CRA creates a simple web app to start with, including most of the required configuration for general Single Page Application needs, whatever your taste: Typescript, CSS preprocessors like SASS or LESS and more.
The app is bound to a single HTML page that, when open, loads the React apps – this provided an incredible Developer Experience (DX) for the development process: errors don’t stop development any more, installing new dependencies doesn’t mean the need to stop and restart, and on and on!
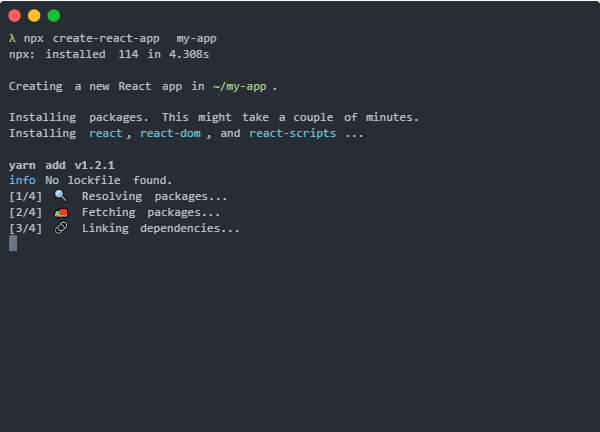
Note that there’s no bundled React library with CRA, nor i18n, state management or other advanced features, so it’s completely up to the React developer to pick the right library to include in their project.
This makes the bootstrapped project less opinionated and means it’s ready to be used to experiment with any React JS library or to quickly prototype a basic app.
The final product of a CRA build is one single HTML page, with JS and CSS files containing the web application bundle. This means that the build folder can be statically deployed anywhere and does not depend on any particular web server.
What if the configuration provided in CRA is still not enough?
In this situation, it is always possible to “eject” from the classic configuration, and customize it. The “eject” task has a major downside, though – losing the ability to upgrade again using the main create-react-app streams.
There are a few projects out there that help with that, like react-app-rewired project, which can be used in these scenarios without a full “eject”, maintaining the ability to upgrade later on.
Would it be possible to have the development experience of this tool on the server side? Could React be used to build a website that loads like static, but is as fast as an SPA when using it? This is the idea that powers Gatsby.
Gatsby
Gatsby is based on React’s open-source framework offering built-in performance, scalability and security. It is the solution created by Facebook to enable the use of ReactJS on the server side, or as it is often referred to, React SSR (React Server Side Rendering).
The product of a React SSR is a plain HTML page, like any other backend rendering technology: the advantage of using React is the “hydrating” process that can make it an SPA on the front-end, as explained below.
The goal of Gatsby is to make the development of websites super performant by leveraging the React developer experience.
Although the website is modelled as an SPA – going as far as to have a routing system in React JS; the Gatsby router – what actually happens is that Gatsby will build both a static website and also an SPA from the material.
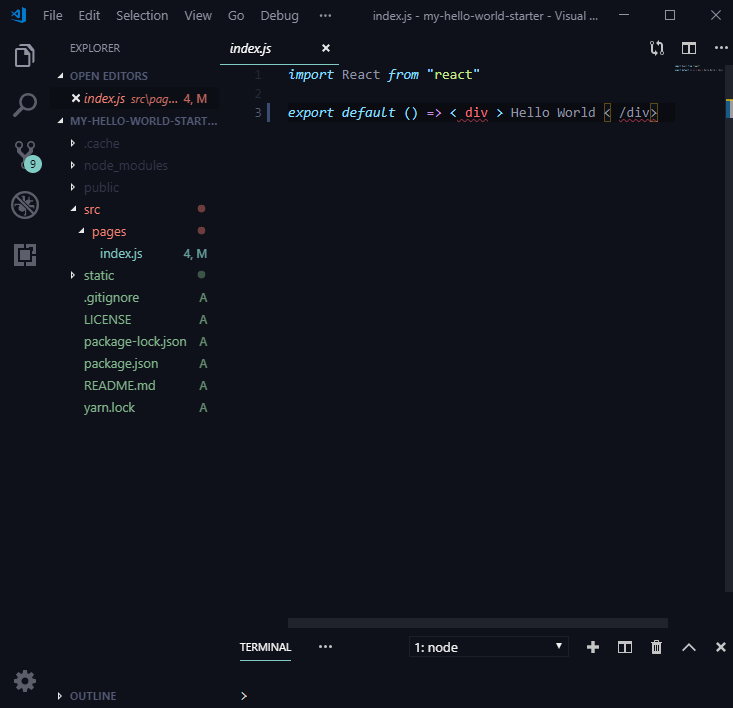
The React SSR version of Gatsby can be seen as a “compile” step for all pages, enabling you to serve them as static content.
Like CRA, Gatsby has many configurations that provide support for Typescript, CSS preprocessors and so on. Gatsby is particularly focused on websites, and it provides an out-of-the-box system based on GraphQL for easy content fetching, with a multitude of plugins for theming and every kind of support.
The reason for building both a static version of the website and an SPA is that this provides an instant website load in a few milliseconds via the static version, then “hydrates” the website with an equivalent React JS SPA to make it interactive.
This means that the user will see the website has an excellent performance on first load, then with one click it behaves as a client app without accessing the servers again.
This sounds awesome, right? In fact, although it’s great, it comes with a built-in limit: the website must be static, so all possible HTML pages must be created in advance. This means that dynamic routes are not possible in websites built with Gatsby.
If we’re talking about the deployable artifact, with Gatsby we’re still building frontend assets: a folder full of HTML pages and JS/CSS scripts. The Gatsby team built a full infrastructure to help optimize the building step for developers, named Gatsby Cloud. The deployment step in Gatsby is straightforward – a simple upload of the folder resources on a web server.
But is it possible to have a real server developed with React SSR that has the possibility of a SPA on the front? The answer to this is Next.js.
Next.js
Next.js is a project to bring ReactJS to the server side, and combine the SPA experience with it. It’s built with ZEIT (now Vercel), with dynamic routes.
Next.js is open source, developed in Node, and it can be used either as a web server or can be integrated into bigger node applications – like Express.js, Hapi, Fastify, etc. – providing React remains the rendering engine.
There are some additional React JS conventions in this tool to bear in mind, because the same app page can be rendered on the server, on the client or in AMP (Accelerated Mobile Pages).
Under the hood of the build version of a Next application, Webpack is used to optimize the final app artifact (while already optimized, the configuration can be extended at will).
Next.js makes the integration between back and front end development in Javascript (or Typescript ) of complex web apps seamless. The build products of Next.js are then a Node.JS app with front end assets for the SPA.
Next.js provides a full React SSR experience with dynamic page rendering. Since Next.js 9.3, a new Static Site Generation feature is available, which aligns Next.js with Gatsby in the static generation market, providing a unique hybrid static and dynamic server rendering tool.
Gatsby.JS vs Next.JS vs CRA
Gatsby.Js and Next.Js are both React frameworks that have been around for some time now and have in common quite a few things.
Fort starters, Gatsby and Next both come with extensive documentation so you don’t have to learn everything from scratch; a basic understanding of React is the only prerequisite.
Also, Gatsby and Next are both SEO-friendly and are used to build high-performance websites especially for their ability to deliver pre-rendered HTML pages.
Finally, both Gatsby and Next are excellent JS frameworks for web development with built-in features such as Hot Reloading, Code Splitting, and Prefetching.
But the similarities end here. Each of them has distinct features that make them stand out from the other. Hence, they are used for fundamentally different purposes.
Peculiarities of GatsbyJS
Gatsby is a modern front-end framework that provides incredibly fast page-loads, as well as features like asset optimization, server-side rendering, data pre-fetching. It combines the best of React, GraphQL, and React-router providing a very Web developer-friendly static site generator.
Gatsby’s static HTML files are hosted on CDNs for faster access so it has not to rely on a server or database to make queries.
Peculiarities of Next.JS
Next.JS is instead used to build dynamic server-side rendered websites that require to interact a lot with the server every time a new request comes to it. To enhance the development experience, it lets you use features like Hot Code Reloading, Single File components, and Automatic Code Splitting.
Because of its semplicity, you can use Next.js effectively and in no time. As a Web developer, you probably won’t need a tutorial to get started. In fact, you don’t have to be an experienced JavaScript developer or code like a pro to use it.
Peculiarities of CRA
CRA is a new project created to simplify the process of getting started with React development. It configures webpack and Babel for you, so all you need to do is install npm and Node. It can be used to develop single-page app (SPA), i.e. websites that do not require refreshing when navigating through pages, hence offering a very fast and fluid browsing experience.
The browser extension usually stores user data locally during the time spent on the page, and is able to create home environments for the user.
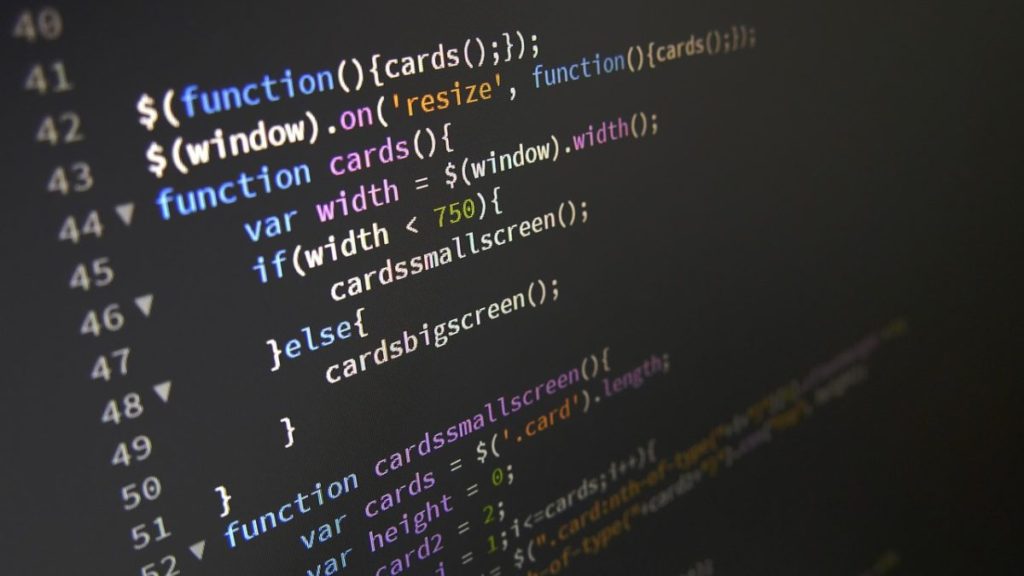
What if I need something different?
The three options discussed here are only the most famous tools in the React JS world with which to start your project, but there are more available that cater to specific niches or tastes, for instance Docusaurus or react-boilerplate project.
Using one of these tools is probably the best bet to start with, but the React community has already managed to pull some magic out of the hat a few times in the past, so feel free to explore what’s out there for new and wonderful discoveries.
Conclusions
It is quite clear that all of these frameworks are great for developing apps with React. To choose one against the other is more a matter of what is your use case going to be for a specific project.
NextJS by Vercel has been growing in popularity and the reason is quite simple. This JS framework does it all. It seems to have taken the best of Gatsby’s and CRA’s functions to offer them either independently or in combination to Web developers. If you have no time for tutorials and like a zero-config approach and out-of-the-box routing, styling, and code-splitting, this might be your best choice.
Recommended article: React Native – Alive and Kicking in 2022