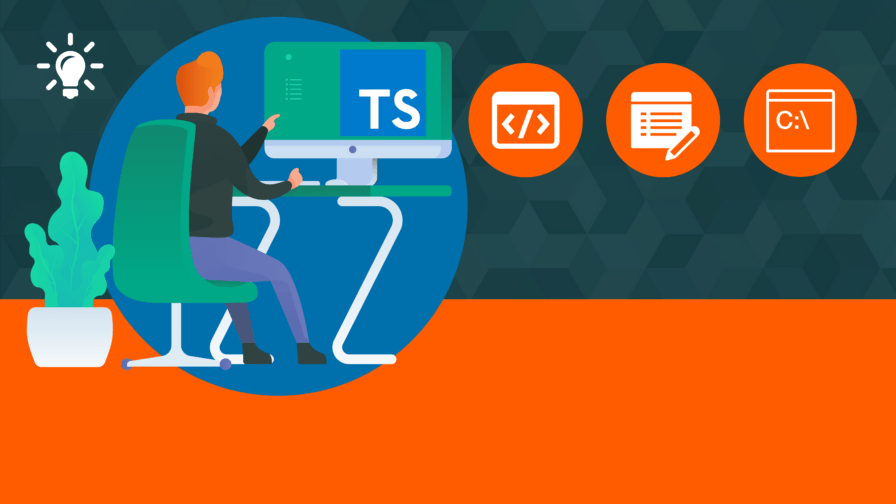
What is TypeScript?
TypeScript is a programming language first developed by Microsoft in 2012. Its main ambition is to improve the productivity of developing complex applications.
It is an open-source language developed as a superset of JavaScript. What this means in simple terms is that any code valid in JavaScript is also valuable for TypeScript.
However, the language introduces optional features like typing or object-oriented programming. To benefit from these features, no library is required. Instead, just use the TypeScript compilation tool to transpile it (compiling the source code of one language into another language) into JavaScript. Thus, the executed code will be a Javascript equivalent of the compiled TypeScript code.
Beyond Javascript and more
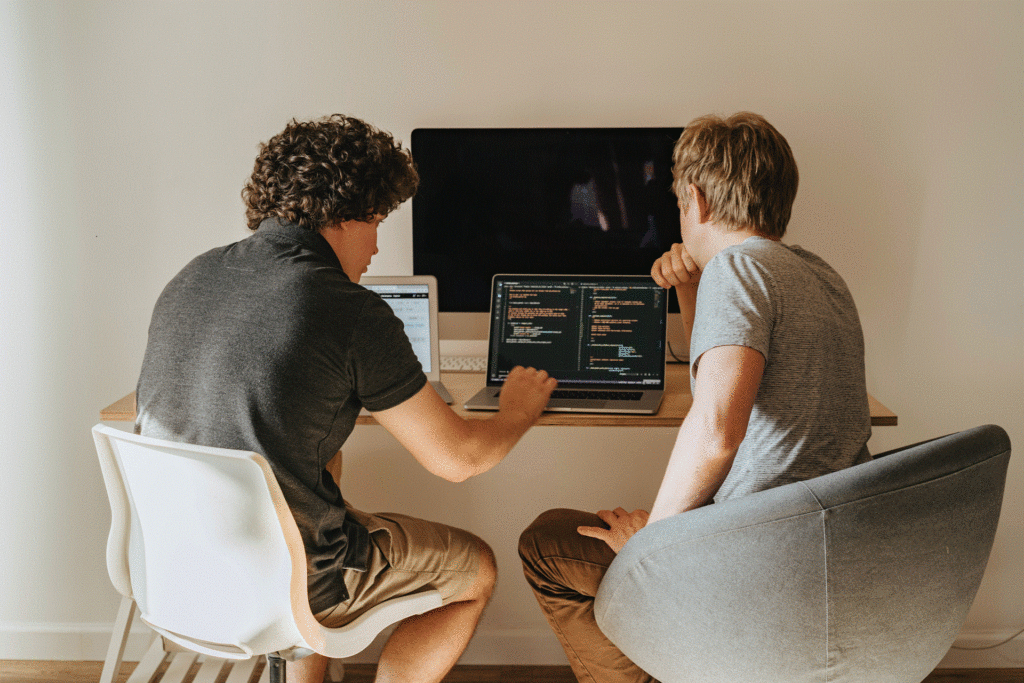
In terms of software development, TypeScript offers many advantages over JavaScript:
- Optional static typing. JavaScript is a dynamically typed language, which means that types are checked, and data type errors are only detected at runtime. This can be very dangerous and can create errors during production. TypeScript introduces optional strong static typing: once declared, a variable does not change its type and can only take specific values.
- Readability. Thanks to the addition of strict types and other elements that make the code more self-expressive, you can see the design intent of the developers who originally wrote the code. This is especially important for distributed teams working on the same project. A code that speaks for itself can compensate for the lack of direct communication between team members.
- IDE support. Type information renders editors and integrated development environments (IDEs) much more useful. They can offer features like code navigation and auto-complete, providing accurate suggestions.
- The power of object-orientation. TypeScript supports Object-Oriented Programming (OOP) concepts such as classes, interfaces, inheritance, etc. The OOP paradigm makes it easier to build well-organized scalable code, and this advantage becomes more apparent as your project grows in size and complexity.
- The support of a talented community. Behind TypeScript is a massive community of very gifted people working day by day to improve the language.
- Integrated support for updated versions of ECMAScript, which is the scripting language that forms the basis of JavaScript.
- ECMAScript defines the standards and novelties of JavaScript. TypeScript takes great care to include all these new features with each update.
Typescript and NodeJS
NodeJS is a runtime environment that enables you to write server-side JavaScript. It has been widely adopted since its release in 2011. Writing server-side JavaScript can be difficult as the code base grows due to the nature of the JavaScript language; dynamic and weakly typed.
Developers using JavaScript from other languages often complain about the lack of strong static characters, but that’s where TypeScript comes in to fill that gap.
TypeScript is a superset of typed JavaScript (optional) that can help build and manage large-scale JavaScript projects. It can be considered JavaScript with additional features like strong static typing, compilation, and object-oriented programming.
Why choose TypeScript for your next project?
When TypeScript was new, some developers were a little reluctant to learn the new skills.
However, over time, many have come to appreciate it. The strength of TypeScript is not felt in the first moments of a project’s life but over the long term.
This language is evolving rapidly, and the developers’ community continues to grow. As a result, new versions are regularly released and make it easier to get started with TypeScript, use it daily, and add even more powerful features. In fact, TypeScript ranks number ten in The PYPL PopularitY of Programming Language Index.
Once comfortable with the language, it’s clear that developers have a better understanding of the code and the libraries used.
But the most important and revolutionary aspect that it brings for front-end developers is auto-completion. Back-end developers should find this amusing because this is something they’ve known for a long time! But for front-end developers, this is one of the main advantages of TypeScript.
It acts as living documentation, allowing tools (IDE) to offer relevant and reliable auto-completion.
Programming and Objects
TypeScript allows you to write JavaScript in a way that’s right for you. It is basically a JavaScript typed superset that collates to raw JavaScript.
To start
Node.js is an open-source, multi-platform runtime environment for server-side JavaScript. Node.js is required to run JavaScript without the need for browser support. Installing TypeScr*pt is straightforward; simply type the following command in the terminal window:
$ npm install -g typescript
In common with other programming languages, TypeScript contains the following data types:
String — A string is a sequence of characters
Integer — An integer data type is a non-decimal number
Float — A float is a number with a comma or a number
Boolean — A Boolean represents TRUE or FALSE.
Array — An array stores multiple values in a single variable.
How to install TypeScript
Before going further, you must install the following tools:
– Vs code: which is currently the IDE par excellence for the language
– Node js: The javascript server will allow us to use the npm tool.
After having installed them following the instructions of their respective sites, you must follow the following installation steps:
Create your project folder. Let’s name it, for example, project_startup
Open your vscode code editor then click File->open folder ->select project_startup
After the npm init command, you will be prompted to set up your project. Press Enter to keep the default configuration.
There are lots of coding examples online for more detail.
Interface
One of TypeScript’s main principles is that type checking concentrates on the shape of values. This is sometimes referred to as “duck typing” or “structural subtyping”. In TypeScript, interfaces have the function of naming these types and are an effective way of defining contracts within the code in addition to contracts with code away from your project.
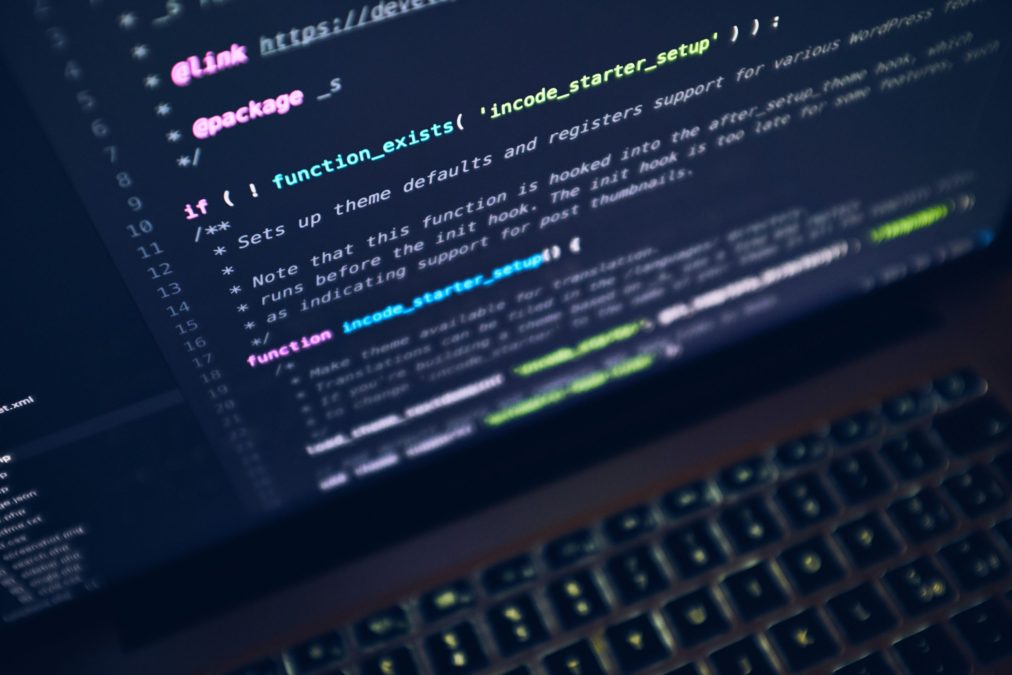
Interfaces and classes are the fundamental parts of object-oriented programming (OOP). For example, TypeScript is an object-oriented JavaScript language that, from ES6 and later, supports OOP features like interface, class, and encapsulation.
Interface is a structure that sets out the contract in your application. It establishes the syntax for classes to follow. Classes derived from an interface must comply with the structure given by their interface. However, the TypeScript compiler does not convert the interface to JavaScript.
Working with large projects
TypeScript was born out of JavaScript’s shortcomings for large-scale application development both at Microsoft and their external customers.
It’s not the only example of technology that has tried to solve a problem with large JavaScript projects. There are also: PureScript, Reason, Elm or ClojureScript.
In general, it does not matter which one you choose. The most important question is whether it meets your needs. Anyway, there are some reasons why TypeScript deserves special attention.
It’s easier to manage your work on Typescript on a large application compared to JavaScript. You can leverage the OOPs fundamentals to simplify your work quite a bit. In addition, the Editors can help you better in TypeScript to minimize bugs due to type mismatches.
Some final thoughts
The advantage of TypeScript, as mentioned above, is, therefore, mainly for developers.
The choice of TypeScript over JavaScript will make it possible to remove certain software anomalies earlier during the development phase and secure the code before going into production. In reality, thanks to stricter rules, the TypeScript language will make it easier to manage and anticipate special cases, which the team would not have thought of spontaneously, by forcing developers to take them into account so that the product code is valid. This saves time in developing features and avoids integrating bugs without realizing them into a program.
TypeScript is also very useful and efficient when the day comes for code redesigns or factorizations. If a code module changes its behaviour, the development team will be automatically alerted wherever this module is used and implemented. Therefore, the team will be more serene and secure in the redesign, thus improving the maintainability of a project over time.
As mentioned before, the code is more readable and easier to understand, makes it easy to onboard new developers to a project, and reduces the cost of entry (which can be quite colossal on large-scale projects).
The advantage also for a start-up will be to attract slightly more experienced and rigorous developers within their company, thus increasing the quality of the applications produced and internal projects.
What should be remembered is that this language makes it easy to do object-oriented programming with javascript. In addition, it improves developer productivity by clearly displaying syntax errors during compilation as if it were a native language.
Want to discover more about TypeScript? Watch the video below!
Recommended articles
Typescript 10 Years After Release
Create an MDX blog with Typescript and Next