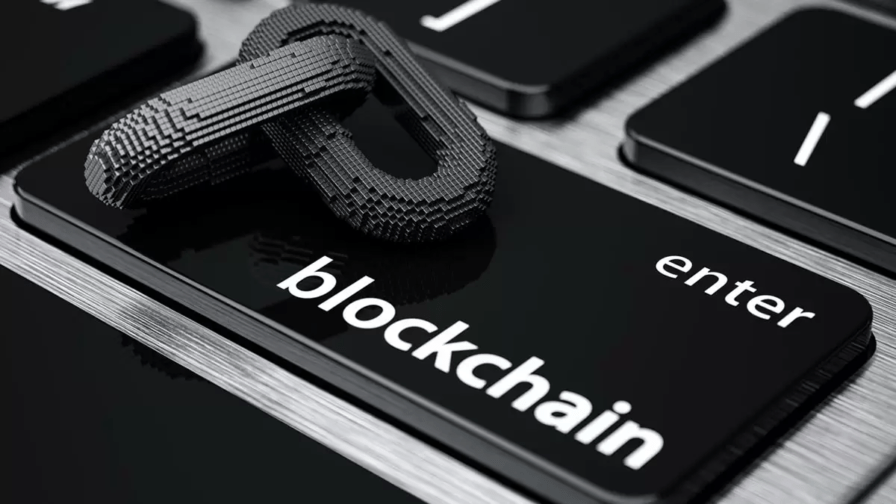
Introduction
In previous articles in this series, we learned how a basic contract is made, and discovered that Ethereum Studio is a simple and easy way to develop and deploy on a testnet. We demonstrated how to build a simple web interface using the Web3.js Javascript framework to handle user interaction via web browser. With that in mind, in this chapter we will go through an example of a web page, and in the following (and final) chapter, we’ll see how web3 is actually implemented in order to connect the smart contract to a web page.
This article is part four of a series on how to create an ERC-20 token. If you want to begin from scratch, please read part one, part two and part three of this series first (here’s part five).
Smart contract: building a simple interface
Web3.js is the Javascript framework that allows users to interact with the Blockchain. To implement a smart contract with an interactive interface, we must build a simple web page.
We begin by creating an HTML file:
<!DOCTYPE html>
<html lang="en">
// here lies the smart contract interface
</html>
Code language: HTML, XML (xml)
Then, we need to configure the metadata in the header. We load jQuery and Web3.js packages:
<head>
<meta charset="utf-8">
<script type="text/javascript" src="https://unpkg.com/jquery@3.3.1/dist/jquery.js"></script>
<script type="text/javascript" src="https://unpkg.com/web3@0.20.5/dist/web3.min.js"></script>
</head>
Code language: HTML, XML (xml)
Then we can actually design the interface. In this example, we are going to create a user interface that has two sections: the first will let users create an address with an attached amount of token, whilst the second will check the balance of an address.
Here is the first section:
<body>
<div id="create" class="tabcontent">
<h2>Create new coins</h2>
<div class="input-group">
<h3>Address</h3>
<input type="text" id="create-address" placeholder="Enter address" />
</div>
<div class="input-group">
<h3>Amount</h3>
<input type="number" id="create-amount" placeholder="Enter amount..." />
</div>
<div class="input-group">
<button class="btn" id="button-create">Send</button>
</div>
</div>
Code language: HTML, XML (xml)
And here is the second section:
<div id="balances" class="tabcontent">
<h2>Check balance of wallet</h2>
<div class="input-group">
<h3>Address</h3>
<input type="text" id="balance-address" placeholder="Enter address..." />
</div>
<div class="input-group">
<button class="btn" id="button-check">Check balance</button>
</div>
</div>
<h1 class="text message">Balance: <span id="message"></span></h1>
</body>
Code language: HTML, XML (xml)
It’s important to note that there’s not even a single script within the HTML code. That’s because the contract connects with the page through a .js file that builds objects from the smart contract.
In the <div>
elements, one can see the id
of every element: these will later be called by the .js core to match the attributes in the smart contract.
Here’s the complete HTML page:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<script type="text/javascript" src="https://unpkg.com/jquery@3.3.1/dist/jquery.js"></script>
<script type="text/javascript" src="https://unpkg.com/web3@0.20.5/dist/web3.min.js"></script>
</head>
<body>
<div id="create" class="tabcontent">
<h2>Create new coins</h2>
<div class="input-group">
<h3>Address</h3>
<input type="text" id="create-address" placeholder="Enter address..." />
</div>
<div class="input-group">
<h3>Amount</h3>
<input type="number" id="create-amount" placeholder="Enter amount..." />
</div>
<div class="input-group">
<button class="btn" id="button-create">Send</button>
</div>
</div>
<div id="balances" class="tabcontent">
<h2>Check balance of wallet</h2>
<div class="input-group">
<h3>Address</h3>
<input type="text" id="balance-address" placeholder="Enter address..." />
</div>
<div class="input-group">
<button class="btn" id="button-check">Check balance</button>
</div>
</div>
<h1 class="text message">Balance: <span id="message"></span></h1>
</body>
</html>
Code language: HTML, XML (xml)
So, to recap:
- The logic of the token is described in the .sol file, in Solidity;
- The token logic is built (initialized) in a .js file, that ‘prepares’ the smart contract to be interactive in a HTML page;
- The HTML page visualizes the objects rendered with the .js file.
Of course, the HTML page can and should be built with a CSS file as well (which can be put in the same folder as the HTML page). Coding the stylesheet of the interface is beyond the scope of this series, so we won’t show it here, but the page can be customized as much as one wants.
So, we have the token logic and we have designed the HTML page. We must now code the .js core file that acts as a bridge between the two. That’s the focus of the final chapter in this series.