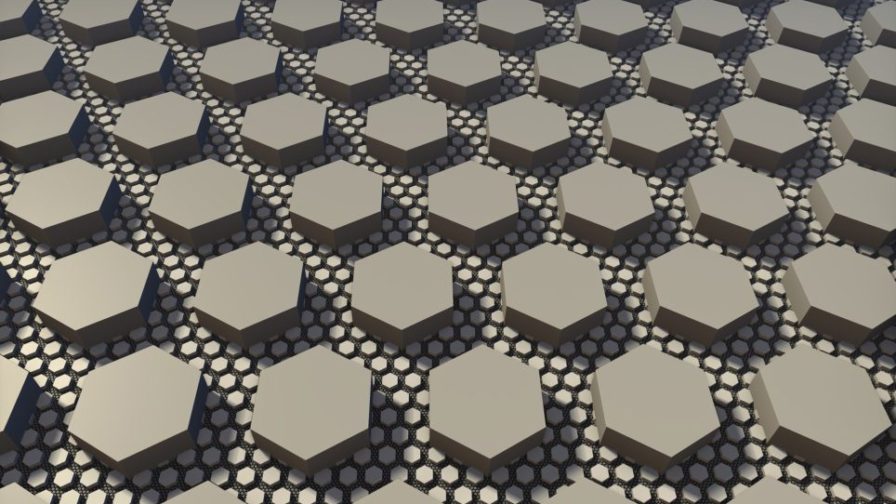
This article is the first in a series (part two, part three, part four and part five) that will teach you how to develop a simple smart contract on the Ethereum Blockchain. Before delving into more technical information, we need to be sure that everyone is on the same page.
This first chapter provides a basic understanding of Blockchain technology and the dynamics of an interactive blockchain such as Ethereum.
What is a Blockchain
A Blockchain is a peer to peer decentralized payment system, first conceived by its creator Satoshi Nakamoto. A Blockchain is a software technology that enables users to send and receive money and/or goods by writing the transactions on a distributed ledger that is accessible to anyone at any time.
In a Blockchain system, consensus on a transaction is reached using a specific protocol. Bitcoin (and later Ethereum) was designed using the Byzantine Fault Tolerance consensus protocol, which resolves agreements in distributed computing and multi-agent systems based on the Byzantine Generals Problem, an extension of the Two Generals Problem.
Goods and assets on a Blockchain are identified through addresses – strings of characters associated with an amount of cryptocurrency (token).
What is Ethereum
Ethereum is defined as Blockchain 2.0 – it’s a Blockchain that lets developers enrich the metadata contained in a transaction in order to build smart applications on the distributed ledger.
Like Bitcoin, Ethereum is a distributed public Blockchain network. Ether (ETH) is the cryptocurrency earned as a reward for the process of ‘mining’, and is used as ‘fuel’ by application developers and users to pay the processing fees of decentralized applications. This fee, called gas, is usually so low that is measured in Gwei. A Gwei is equivalent to 1 x 10^9 ETH.
It’s important to understand the value of a single Gwei: gas is very like the instruction clock of a CPU. Ethereum is defined as the world’s global operating system, and that definition is not only metaphorical, but also practical. When coding a smart contract, every instruction is translated into a lower-level language, the Ethereum Virtual Machine (EVM). The EVM is the equivalent of the Java Virtual Machine: it translates the abstract instructions into instruction that can be processed by the machine. In the case of Ethereum, the machine is not a single computer, but a network of computers that validates each instruction and processes the whole program, updating the state of the function, i.e. the data associated with the smart contract.
What is a token
As per the universal definition, a token is the representation of a real asset or entity in a digital system. In a decentralized peer to peer distributed system such as the Blockchain, a token is the digital representation of either virtual currency or a digital asset in the distributed ledger that can be owned, transferred and modified by users who access the platform associated with the token. Such platforms are called DApp (Decentralized Application), and can be seen as decentralized versions of a web application. Examples of Decentralized Applications include games like cryptokitties.co or cheezewizards.com, and other platform types, such as compound.finance or ethlend.io.
Let’s take the example of Cryptokitties: the application runs on Ethereum and lets users trade Crypto Collectibles (like trading cards) for Ether. Every token represents a cryptokitty. Users can trade a collectible and own the exclusive right to that token, held in their own wallet. This type of token is called non-fungible; each token is unique but every token has the same characteristics – every kitty has two eyes, a tail, a body in a specific color, but each kitty has these in a different combination. One token may be more expensive than another, and this dynamic creates a specific market for every token. Non-fungible tokens are not in the scope of this series, but this brief overview is necessary to illustrate the following.
Fungible tokens, in contrast, are ‘simple’ tokens that can be seen as money: every token is equal to every other other, and the only difference between those held by two users lies in the amount of tokens held by each individual. Ether (ETH) is a fungible token: every user can trade Ether, and all Ether tokens have the same value, i.e., the value of Ether. In this series, we will discover how to create a token like ETH on the Ethereum blockchain.
What is a smart contract
Put simply, a smart contract is a computer protocol intended to digitally facilitate, verify, or enforce the negotiation or performance of a contract. Smart contracts allow credible transactions to be performed without involving third parties.
As of today, Ethereum is the most popular Blockchain used to run smart contracts.
The following is a ‘Hello World’ smart contract:
pragma solidity >=0.4.0 <0.7.0; contract SimpleStorage { uint storedData; function set(uint x) public { storedData = x; } function get() public view returns (uint) { return storedData; } }
As is clear from the first line, the first big thing potential users should know about Ethereum smart contracts is that they are written in a whole new language – Solidity.
Solidity is an object-oriented, high-level language influenced by C++, Python and Javascript. It is designed to target the Ethereum Virtual Machine.
Statically typed, Solidity supports inheritance, libraries and complex user-defined types among other features.
This basic smart contract is designed to simply return a variable stored in the main function, but it’s already a prime example of how a smart contract works in its entirety: there are functions and there is state.
- Functions work exactly as a function would in any other object-oriented language. These are the operations that a contract can perform, getting data as input and producing an output to return.
- State is the state of the contract in the Ethereum network, with a specific address on the blockchain.
storedData is the main (and only) variable of the contract of type uint, and set(uint x) public is the main method that sets the variable in the state of the contract. The get() function returns the variable as the output of the whole contract.
We can think of this basic smart contract as a simple class with get and set functions. One subtle difference that should be noted is the absence of the prefix this. in the set function, as it is not needed in Solidity.
This smart contract can store and show a simple number for general viewing on the public Blockchain; any user could use this contract to set another number that will then overwrite the previous number, but the contract will always store its state in the blockchain, so it’s possible to see every previous state (i.e. number set) by looking for previous transactions associated with the address of the contract.
With all the necessary elements outlined, we can dive into how to write a smart contract, as well as how to design a smart contract that is functional within the context of a decentralized infrastructure.
Stay up to date and follow us to learn more about Blockchain!